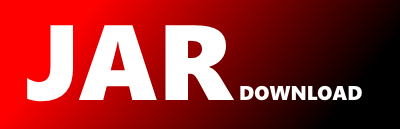
com.svix.models.TemplateOut Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of svix Show documentation
Show all versions of svix Show documentation
Svix webhooks API client and webhook verification library
/*
* Svix API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.1.1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.svix.models;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.svix.models.TransformationTemplateKind;
import java.io.IOException;
import java.net.URI;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.LinkedHashSet;
import java.util.Set;
import org.openapitools.jackson.nullable.JsonNullable;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.svix.internal.JSON;
/**
* TemplateOut
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-12-12T23:42:33.364995Z[Etc/UTC]", comments = "Generator version: 7.9.0")
public class TemplateOut {
public static final String SERIALIZED_NAME_CREATED_AT = "createdAt";
@SerializedName(SERIALIZED_NAME_CREATED_AT)
private OffsetDateTime createdAt;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_FEATURE_FLAG = "featureFlag";
@SerializedName(SERIALIZED_NAME_FEATURE_FLAG)
private String featureFlag;
public static final String SERIALIZED_NAME_FILTER_TYPES = "filterTypes";
@SerializedName(SERIALIZED_NAME_FILTER_TYPES)
private Set filterTypes;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_INSTRUCTIONS = "instructions";
@SerializedName(SERIALIZED_NAME_INSTRUCTIONS)
private String instructions;
public static final String SERIALIZED_NAME_INSTRUCTIONS_LINK = "instructionsLink";
@SerializedName(SERIALIZED_NAME_INSTRUCTIONS_LINK)
private URI instructionsLink;
public static final String SERIALIZED_NAME_KIND = "kind";
@SerializedName(SERIALIZED_NAME_KIND)
private TransformationTemplateKind kind;
public static final String SERIALIZED_NAME_LOGO = "logo";
@SerializedName(SERIALIZED_NAME_LOGO)
private URI logo;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_ORG_ID = "orgId";
@SerializedName(SERIALIZED_NAME_ORG_ID)
private String orgId;
public static final String SERIALIZED_NAME_TRANSFORMATION = "transformation";
@SerializedName(SERIALIZED_NAME_TRANSFORMATION)
private String transformation;
public static final String SERIALIZED_NAME_UPDATED_AT = "updatedAt";
@SerializedName(SERIALIZED_NAME_UPDATED_AT)
private OffsetDateTime updatedAt;
public TemplateOut() {
}
public TemplateOut createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Get createdAt
* @return createdAt
*/
@javax.annotation.Nonnull
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public TemplateOut description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nonnull
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public TemplateOut featureFlag(String featureFlag) {
this.featureFlag = featureFlag;
return this;
}
/**
* Get featureFlag
* @return featureFlag
*/
@javax.annotation.Nullable
public String getFeatureFlag() {
return featureFlag;
}
public void setFeatureFlag(String featureFlag) {
this.featureFlag = featureFlag;
}
public TemplateOut filterTypes(Set filterTypes) {
this.filterTypes = filterTypes;
return this;
}
public TemplateOut addFilterTypesItem(String filterTypesItem) {
if (this.filterTypes == null) {
this.filterTypes = new LinkedHashSet<>();
}
this.filterTypes.add(filterTypesItem);
return this;
}
/**
* Get filterTypes
* @return filterTypes
*/
@javax.annotation.Nullable
public Set getFilterTypes() {
return filterTypes;
}
public void setFilterTypes(Set filterTypes) {
this.filterTypes = filterTypes;
}
public TemplateOut id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nonnull
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public TemplateOut instructions(String instructions) {
this.instructions = instructions;
return this;
}
/**
* Get instructions
* @return instructions
*/
@javax.annotation.Nonnull
public String getInstructions() {
return instructions;
}
public void setInstructions(String instructions) {
this.instructions = instructions;
}
public TemplateOut instructionsLink(URI instructionsLink) {
this.instructionsLink = instructionsLink;
return this;
}
/**
* Get instructionsLink
* @return instructionsLink
*/
@javax.annotation.Nullable
public URI getInstructionsLink() {
return instructionsLink;
}
public void setInstructionsLink(URI instructionsLink) {
this.instructionsLink = instructionsLink;
}
public TemplateOut kind(TransformationTemplateKind kind) {
this.kind = kind;
return this;
}
/**
* Get kind
* @return kind
*/
@javax.annotation.Nonnull
public TransformationTemplateKind getKind() {
return kind;
}
public void setKind(TransformationTemplateKind kind) {
this.kind = kind;
}
public TemplateOut logo(URI logo) {
this.logo = logo;
return this;
}
/**
* Get logo
* @return logo
*/
@javax.annotation.Nonnull
public URI getLogo() {
return logo;
}
public void setLogo(URI logo) {
this.logo = logo;
}
public TemplateOut name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nonnull
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public TemplateOut orgId(String orgId) {
this.orgId = orgId;
return this;
}
/**
* Get orgId
* @return orgId
*/
@javax.annotation.Nonnull
public String getOrgId() {
return orgId;
}
public void setOrgId(String orgId) {
this.orgId = orgId;
}
public TemplateOut transformation(String transformation) {
this.transformation = transformation;
return this;
}
/**
* Get transformation
* @return transformation
*/
@javax.annotation.Nonnull
public String getTransformation() {
return transformation;
}
public void setTransformation(String transformation) {
this.transformation = transformation;
}
public TemplateOut updatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Get updatedAt
* @return updatedAt
*/
@javax.annotation.Nonnull
public OffsetDateTime getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TemplateOut templateOut = (TemplateOut) o;
return Objects.equals(this.createdAt, templateOut.createdAt) &&
Objects.equals(this.description, templateOut.description) &&
Objects.equals(this.featureFlag, templateOut.featureFlag) &&
Objects.equals(this.filterTypes, templateOut.filterTypes) &&
Objects.equals(this.id, templateOut.id) &&
Objects.equals(this.instructions, templateOut.instructions) &&
Objects.equals(this.instructionsLink, templateOut.instructionsLink) &&
Objects.equals(this.kind, templateOut.kind) &&
Objects.equals(this.logo, templateOut.logo) &&
Objects.equals(this.name, templateOut.name) &&
Objects.equals(this.orgId, templateOut.orgId) &&
Objects.equals(this.transformation, templateOut.transformation) &&
Objects.equals(this.updatedAt, templateOut.updatedAt);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(createdAt, description, featureFlag, filterTypes, id, instructions, instructionsLink, kind, logo, name, orgId, transformation, updatedAt);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TemplateOut {\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" featureFlag: ").append(toIndentedString(featureFlag)).append("\n");
sb.append(" filterTypes: ").append(toIndentedString(filterTypes)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" instructions: ").append(toIndentedString(instructions)).append("\n");
sb.append(" instructionsLink: ").append(toIndentedString(instructionsLink)).append("\n");
sb.append(" kind: ").append(toIndentedString(kind)).append("\n");
sb.append(" logo: ").append(toIndentedString(logo)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" orgId: ").append(toIndentedString(orgId)).append("\n");
sb.append(" transformation: ").append(toIndentedString(transformation)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("createdAt");
openapiFields.add("description");
openapiFields.add("featureFlag");
openapiFields.add("filterTypes");
openapiFields.add("id");
openapiFields.add("instructions");
openapiFields.add("instructionsLink");
openapiFields.add("kind");
openapiFields.add("logo");
openapiFields.add("name");
openapiFields.add("orgId");
openapiFields.add("transformation");
openapiFields.add("updatedAt");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("createdAt");
openapiRequiredFields.add("description");
openapiRequiredFields.add("id");
openapiRequiredFields.add("instructions");
openapiRequiredFields.add("kind");
openapiRequiredFields.add("logo");
openapiRequiredFields.add("name");
openapiRequiredFields.add("orgId");
openapiRequiredFields.add("transformation");
openapiRequiredFields.add("updatedAt");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to TemplateOut
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!TemplateOut.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in TemplateOut is not found in the empty JSON string", TemplateOut.openapiRequiredFields.toString()));
}
}
Set> entries = jsonElement.getAsJsonObject().entrySet();
// check to see if the JSON string contains additional fields
for (Map.Entry entry : entries) {
if (!TemplateOut.openapiFields.contains(entry.getKey())) {
throw new IllegalArgumentException(String.format("The field `%s` in the JSON string is not defined in the `TemplateOut` properties. JSON: %s", entry.getKey(), jsonElement.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : TemplateOut.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if (!jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("featureFlag") != null && !jsonObj.get("featureFlag").isJsonNull()) && !jsonObj.get("featureFlag").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `featureFlag` to be a primitive type in the JSON string but got `%s`", jsonObj.get("featureFlag").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("filterTypes") != null && !jsonObj.get("filterTypes").isJsonNull() && !jsonObj.get("filterTypes").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `filterTypes` to be an array in the JSON string but got `%s`", jsonObj.get("filterTypes").toString()));
}
if (!jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if (!jsonObj.get("instructions").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `instructions` to be a primitive type in the JSON string but got `%s`", jsonObj.get("instructions").toString()));
}
if ((jsonObj.get("instructionsLink") != null && !jsonObj.get("instructionsLink").isJsonNull()) && !jsonObj.get("instructionsLink").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `instructionsLink` to be a primitive type in the JSON string but got `%s`", jsonObj.get("instructionsLink").toString()));
}
// validate the required field `kind`
TransformationTemplateKind.validateJsonElement(jsonObj.get("kind"));
if (!jsonObj.get("logo").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `logo` to be a primitive type in the JSON string but got `%s`", jsonObj.get("logo").toString()));
}
if (!jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if (!jsonObj.get("orgId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `orgId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("orgId").toString()));
}
if (!jsonObj.get("transformation").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `transformation` to be a primitive type in the JSON string but got `%s`", jsonObj.get("transformation").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!TemplateOut.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'TemplateOut' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(TemplateOut.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, TemplateOut value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
elementAdapter.write(out, obj);
}
@Override
public TemplateOut read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
return thisAdapter.fromJsonTree(jsonElement);
}
}.nullSafe();
}
}
/**
* Create an instance of TemplateOut given an JSON string
*
* @param jsonString JSON string
* @return An instance of TemplateOut
* @throws IOException if the JSON string is invalid with respect to TemplateOut
*/
public static TemplateOut fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, TemplateOut.class);
}
/**
* Convert an instance of TemplateOut to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy