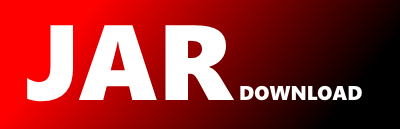
com.swiftmq.tools.collection.ExpandableList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swiftmq-client Show documentation
Show all versions of swiftmq-client Show documentation
Client for SwiftMQ Messaging System with JMS, AMQP 1.0 and file transfer over JMS.
The newest version!
package com.swiftmq.tools.collection;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.Queue;
public class ExpandableList {
protected final List list;
protected final Queue freeIndexes;
public ExpandableList() {
this.list = new ArrayList<>();
this.freeIndexes = new LinkedList<>();
}
public int add(T element) {
Integer freeIndex = freeIndexes.poll();
if (freeIndex != null) {
list.set(freeIndex, element);
} else {
freeIndex = list.size();
list.add(element);
}
return freeIndex;
}
public T get(int index) {
return list.get(index);
}
public void remove(int index) {
list.set(index, null);
freeIndexes.offer(index);
}
public int indexOf(T element) {
return list.indexOf(element);
}
public int size() {
return list.size();
}
public void clear() {
list.clear();
freeIndexes.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy