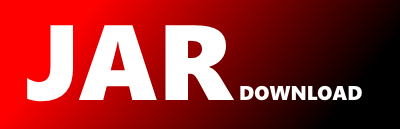
io.swagger.client.model.CardRequest Maven / Gradle / Ivy
/*
* Swirepay
* Swirepay REST APIs' are resource-oriented URLs that accept JSON-encoded request bodies, return JSON-encoded responses, and use standard HTTP response codes, authentication, and verbs. You can use the Swirepay API in test mode, which does not affect your live data or interact with the banking networks. The `API key` you use to authenticate the request determines whether the request is live mode or test mode.
*
* OpenAPI spec version: 1.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
/**
* CardRequest
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2020-12-16T16:28:53.447+05:30[Asia/Kolkata]")
public class CardRequest {
@SerializedName("name")
private String name = null;
@SerializedName("number")
private String number = null;
@SerializedName("scheme")
private String scheme = null;
@SerializedName("cvv")
private String cvv = null;
@SerializedName("expiryMonth")
private String expiryMonth = null;
@SerializedName("expiryYear")
private String expiryYear = null;
public CardRequest name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@Schema(example = "Sample Customer", description = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public CardRequest number(String number) {
this.number = number;
return this;
}
/**
* Get number
* @return number
**/
@Schema(example = "4242424242424242", description = "")
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public CardRequest scheme(String scheme) {
this.scheme = scheme;
return this;
}
/**
* Get scheme
* @return scheme
**/
@Schema(example = "VISA", description = "")
public String getScheme() {
return scheme;
}
public void setScheme(String scheme) {
this.scheme = scheme;
}
public CardRequest cvv(String cvv) {
this.cvv = cvv;
return this;
}
/**
* Get cvv
* @return cvv
**/
@Schema(example = "123", description = "")
public String getCvv() {
return cvv;
}
public void setCvv(String cvv) {
this.cvv = cvv;
}
public CardRequest expiryMonth(String expiryMonth) {
this.expiryMonth = expiryMonth;
return this;
}
/**
* Get expiryMonth
* @return expiryMonth
**/
@Schema(example = "08", description = "")
public String getExpiryMonth() {
return expiryMonth;
}
public void setExpiryMonth(String expiryMonth) {
this.expiryMonth = expiryMonth;
}
public CardRequest expiryYear(String expiryYear) {
this.expiryYear = expiryYear;
return this;
}
/**
* Get expiryYear
* @return expiryYear
**/
@Schema(example = "2024", description = "")
public String getExpiryYear() {
return expiryYear;
}
public void setExpiryYear(String expiryYear) {
this.expiryYear = expiryYear;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CardRequest cardRequest = (CardRequest) o;
return Objects.equals(this.name, cardRequest.name) &&
Objects.equals(this.number, cardRequest.number) &&
Objects.equals(this.scheme, cardRequest.scheme) &&
Objects.equals(this.cvv, cardRequest.cvv) &&
Objects.equals(this.expiryMonth, cardRequest.expiryMonth) &&
Objects.equals(this.expiryYear, cardRequest.expiryYear);
}
@Override
public int hashCode() {
return Objects.hash(name, number, scheme, cvv, expiryMonth, expiryYear);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CardRequest {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" scheme: ").append(toIndentedString(scheme)).append("\n");
sb.append(" cvv: ").append(toIndentedString(cvv)).append("\n");
sb.append(" expiryMonth: ").append(toIndentedString(expiryMonth)).append("\n");
sb.append(" expiryYear: ").append(toIndentedString(expiryYear)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy