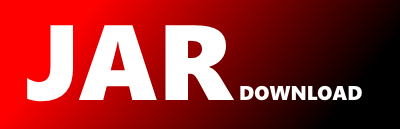
com.swoval.files.FileCachePathWatcher Maven / Gradle / Ivy
package com.swoval.files;
import static com.swoval.functional.Filters.AllPass;
import com.swoval.files.FileTreeDataViews.Entry;
import java.io.IOException;
import java.nio.file.Path;
import java.util.Iterator;
class FileCachePathWatcher implements AutoCloseable {
private final SymlinkWatcher symlinkWatcher;
private final PathWatcher pathWatcher;
private final FileCacheDirectoryTree tree;
FileCachePathWatcher(
final FileCacheDirectoryTree tree, final PathWatcher pathWatcher) {
this.symlinkWatcher = tree.symlinkWatcher;
this.pathWatcher = pathWatcher;
this.tree = tree;
}
boolean register(final Path path, final int maxDepth) throws IOException {
final CachedDirectory dir = tree.register(path, maxDepth, pathWatcher);
if (dir != null && symlinkWatcher != null) {
if (dir.getEntry().isSymbolicLink()) {
symlinkWatcher.addSymlink(path, maxDepth);
}
final Iterator> it = dir.listEntries(dir.getMaxDepth(), AllPass).iterator();
while (it.hasNext()) {
final FileTreeDataViews.Entry entry = it.next();
if (entry.isSymbolicLink()) {
final int depth = path.relativize(entry.getPath()).getNameCount();
symlinkWatcher.addSymlink(
entry.getPath(), maxDepth == Integer.MAX_VALUE ? maxDepth : maxDepth - depth);
}
}
}
return dir != null;
}
void unregister(final Path path) {
tree.unregister(path);
pathWatcher.unregister(path);
}
public void close() {
pathWatcher.close();
if (symlinkWatcher != null) symlinkWatcher.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy