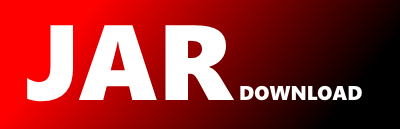
com.swoval.files.FileCachePathWatcher Maven / Gradle / Ivy
package com.swoval.files;
import static com.swoval.functional.Filters.AllPass;
import com.swoval.files.FileTreeDataViews.Entry;
import java.io.IOException;
import java.nio.file.Path;
import java.util.Iterator;
class FileCachePathWatcher implements AutoCloseable {
private final SymlinkWatcher symlinkWatcher;
private final PathWatcher pathWatcher;
private final FileCacheDirectoryTree tree;
FileCachePathWatcher(
final FileCacheDirectoryTree tree, final PathWatcher pathWatcher) {
this.symlinkWatcher = tree.symlinkWatcher;
this.pathWatcher = pathWatcher;
this.tree = tree;
}
boolean register(final Path path, final int maxDepth) throws IOException {
final Path absolutePath = path.isAbsolute() ? path : path.toAbsolutePath();
final CachedDirectory dir = tree.register(absolutePath, maxDepth, pathWatcher);
if (dir != null && symlinkWatcher != null) {
if (dir.getEntry().getTypedPath().isSymbolicLink()) {
symlinkWatcher.addSymlink(absolutePath, maxDepth);
}
final Iterator> it = dir.listEntries(dir.getMaxDepth(), AllPass).iterator();
while (it.hasNext()) {
final FileTreeDataViews.Entry entry = it.next();
if (entry.getTypedPath().isSymbolicLink()) {
final int depth = absolutePath.relativize(entry.getTypedPath().getPath()).getNameCount();
symlinkWatcher.addSymlink(
entry.getTypedPath().getPath(),
maxDepth == Integer.MAX_VALUE ? maxDepth : maxDepth - depth);
}
}
}
return dir != null;
}
void unregister(final Path path) {
final Path absolutePath = path.isAbsolute() ? path : path.toAbsolutePath();
tree.unregister(absolutePath);
pathWatcher.unregister(absolutePath);
}
public void close() {
pathWatcher.close();
if (symlinkWatcher != null) symlinkWatcher.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy