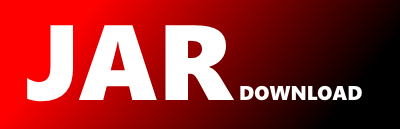
com.swoval.files.MapOps Maven / Gradle / Ivy
package com.swoval.files;
import static java.util.Map.Entry;
import com.swoval.files.FileTreeDataViews.CacheObserver;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* Provides a utility method for diffing two maps of directory entries. It is not in {@link
* CachedDirectoryImpl} because of a name class with java.util.Map.Entry and
* com.swoval.files.CachedDirectory.Entry that breaks code-gen.
*/
class MapOps {
private MapOps() {}
static void diffDirectoryEntries(
final List> oldEntries,
final List> newEntries,
final CacheObserver cacheObserver) {
final Map> oldMap = new HashMap<>();
final Iterator> oldIterator = oldEntries.iterator();
while (oldIterator.hasNext()) {
final FileTreeDataViews.Entry entry = oldIterator.next();
oldMap.put(entry.getTypedPath().getPath(), entry);
}
final Map> newMap = new HashMap<>();
final Iterator> newIterator = newEntries.iterator();
while (newIterator.hasNext()) {
final FileTreeDataViews.Entry entry = newIterator.next();
newMap.put(entry.getTypedPath().getPath(), entry);
}
diffDirectoryEntries(oldMap, newMap, cacheObserver);
}
static void diffDirectoryEntries(
final Map> oldMap,
final Map> newMap,
final CacheObserver cacheObserver) {
final Iterator>> newIterator =
new ArrayList<>(newMap.entrySet()).iterator();
final Iterator>> oldIterator =
new ArrayList<>(oldMap.entrySet()).iterator();
while (newIterator.hasNext()) {
final Entry> entry = newIterator.next();
final FileTreeDataViews.Entry oldValue = oldMap.get(entry.getKey());
if (oldValue != null) {
cacheObserver.onUpdate(oldValue, entry.getValue());
} else {
cacheObserver.onCreate(entry.getValue());
}
}
while (oldIterator.hasNext()) {
final Entry> entry = oldIterator.next();
if (!newMap.containsKey(entry.getKey())) {
cacheObserver.onDelete(entry.getValue());
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy