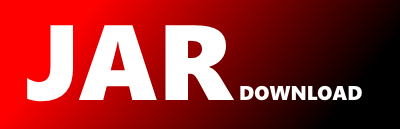
com.symphony.oss.models.fundmental.canon.ObjectsObjectHashVersionsGetHttpRequest Maven / Gradle / Ivy
/**
* GENERATED CODE - DO NOT EDIT OR CHECK IN TO SOURCE CODE CONTROL
*
* Copyright 2020 Symphony Communication Services, LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*----------------------------------------------------------------------------------------------------
* Generated from
* Input source file:/Users/bruce/symphony/git-SymphonyOSF/oss-models/target/checkout/fundamental-model/src/main/resources/canon/fundamental.json
* Template groupId org.symphonyoss.s2.canon
* artifactId canon-template-java
* Template name template/java/Operation/${parent.camelCapitalizedName}${camelCapitalizedName}HttpRequest.java.ftl
* At 2020-01-28 16:55:36 GMT
*----------------------------------------------------------------------------------------------------
*/
package com.symphony.oss.models.fundmental.canon;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.nio.charset.StandardCharsets;
import java.util.LinkedList;
import java.util.List;
import javax.annotation.concurrent.Immutable;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpUriRequest;
import org.apache.http.client.methods.RequestBuilder;
import org.apache.http.client.utils.URLEncodedUtils;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.symphonyoss.s2.common.dom.json.ImmutableJsonList;
import org.symphonyoss.s2.common.dom.json.ImmutableJsonSet;
import org.symphonyoss.s2.canon.runtime.IEntity;
import org.symphonyoss.s2.canon.runtime.JsonArrayParser;
import org.symphonyoss.s2.canon.runtime.exception.BadRequestException;
import org.symphonyoss.s2.canon.runtime.exception.PermissionDeniedException;
import org.symphonyoss.s2.canon.runtime.exception.ServerErrorException;
import org.symphonyoss.s2.common.fault.TransactionFault;
import org.symphonyoss.s2.canon.runtime.http.ParameterLocation;
import org.symphonyoss.s2.canon.runtime.http.IRequestContext;
import org.symphonyoss.s2.canon.runtime.http.client.HttpParameter;
import org.symphonyoss.s2.canon.runtime.http.client.IAuthenticationProvider;
import org.symphonyoss.s2.common.hash.Hash;
import com.symphony.oss.models.fundamental.canon.facade.*;
import com.symphony.oss.models.crypto.canon.facade.*;
import com.symphony.oss.models.crypto.canon.*;
import com.symphony.oss.models.core.canon.facade.*;
import com.symphony.oss.models.core.canon.*;
@Immutable
public class ObjectsObjectHashVersionsGetHttpRequest extends ObjectsObjectHashVersionsGetHttpRequestOrBuilder
{
private static String RESPONSE_TYPE_ID = "com.symphony.s2.model.fundamental.PageOfFundamentalObject";
private final Integer limit_;
private final Boolean scanForwards_;
private final String after_;
private final Hash objectHash_;
private final HttpUriRequest canonRequest_;
private IPageOfFundamentalObject canonResult_;
public ObjectsObjectHashVersionsGetHttpRequest(ObjectsObjectHashVersionsGetHttpRequestOrBuilder other)
{
super(other.getCanonClient());
limit_ = other.getLimit();
scanForwards_ = other.getScanForwards();
after_ = other.getAfter();
objectHash_ = other.getObjectHash();
RequestBuilder builder = RequestBuilder.get()
.setUri(
String.format("%s/objects/%s/versions",
getCanonClient().getUri(),
asString(Hash.toImmutableByteArray(objectHash_))
)
);
IAuthenticationProvider auth = getCanonClient().getAuthenticationProvider();
if(auth != null)
auth.authenticate(builder);
// op parameter Parameter name=limit, required=false, localtion=Query
// op parameter Parameter name=scanForwards, required=false, localtion=Query
// op parameter Parameter name=after, required=false, localtion=Query
// parameter Parameter name=limit, required=false, localtion=Query
if(limit_ != null)
builder.addParameter("limit", asString(limit_));
// parameter Parameter name=scanForwards, required=false, localtion=Query
if(scanForwards_ != null)
builder.addParameter("scanForwards", asString(scanForwards_));
// parameter Parameter name=after, required=false, localtion=Query
if(after_ != null)
builder.addParameter("after", asString(after_));
canonRequest_ = builder.build();
}
@Override
public Integer getLimit()
{
return limit_;
}
@Override
public Boolean getScanForwards()
{
return scanForwards_;
}
@Override
public String getAfter()
{
return after_;
}
@Override
public Hash getObjectHash()
{
return objectHash_;
}
/**
* Execute the request.
*
* @param httpClient An HTTP client to use.
*
* @return A @Nonnull IPageOfFundamentalObject.
*
* @throws TransactionFault If the request fails for technical reasons.
* @throws BadRequestException If the request fails due to bad inputs.
* @throws PermissionDeniedException If the request fails due to authentication or authorization problems.
* @throws ServerErrorException If the request fails due to an unexpected server side error.
*/
public @Nonnull IPageOfFundamentalObject execute(CloseableHttpClient httpClient) throws TransactionFault, PermissionDeniedException, BadRequestException, ServerErrorException
{
CloseableHttpResponse response = null;
try
{
response = httpClient.execute(canonRequest_);
validateResponse(response);
HttpEntity entity = response.getEntity();
JsonArrayParser arrayParser = new JsonArrayParser()
{
@Override
protected void handle(String input)
{
IPageOfFundamentalObject result = getCanonClient().getRegistry().parseOne(new StringReader(input), RESPONSE_TYPE_ID, IPageOfFundamentalObject.class);
canonResult_ = result;
}
};
InputStream in = entity.getContent();
byte[] buf = new byte[1024];
try
{
int nbytes;
while((nbytes= in.read(buf)) != -1)
{
arrayParser.process(buf, nbytes);
}
}
finally
{
arrayParser.close();
in.close();
}
}
catch (IOException e)
{
throw new TransactionFault(e);
}
finally
{
try
{
if(response != null)
response.close();
}
catch (IOException e)
{
throw new TransactionFault(e);
}
}
return canonResult_;
}
}
/*----------------------------------------------------------------------------------------------------
* End of template template/java/Operation/${parent.camelCapitalizedName}${camelCapitalizedName}HttpRequest.java.ftl
* End of code generation
*------------------------------------------------------------------------------------------------- */
© 2015 - 2025 Weber Informatics LLC | Privacy Policy