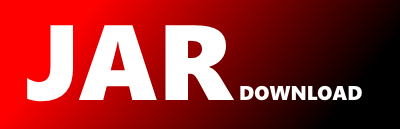
com.symphony.oss.models.fundmental.canon.SecurityContextMemberEntity Maven / Gradle / Ivy
/**
* GENERATED CODE - DO NOT EDIT OR CHECK IN TO SOURCE CODE CONTROL
*
* Copyright 2020 Symphony Communication Services, LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*----------------------------------------------------------------------------------------------------
* Generated from
* Input source file:/Users/bruce/symphony/git-SymphonyOSF/oss-models/target/checkout/fundamental-model/src/main/resources/canon/fundamental.json
* Template groupId org.symphonyoss.s2.canon
* artifactId canon-template-java
* Template name template/java/Object/_Entity.java.ftl
* At 2020-01-28 16:55:36 GMT
*----------------------------------------------------------------------------------------------------
*/
package com.symphony.oss.models.fundmental.canon;
import javax.annotation.Nullable;
import javax.annotation.concurrent.Immutable;
import org.symphonyoss.s2.common.immutable.ImmutableByteArray;
import org.symphonyoss.s2.canon.runtime.IEntity;
import org.symphonyoss.s2.canon.runtime.IModelRegistry;
import org.symphonyoss.s2.canon.runtime.CanonRuntime;
import org.symphonyoss.s2.canon.runtime.Entity;
import org.symphonyoss.s2.canon.runtime.EntityBuilder;
import org.symphonyoss.s2.canon.runtime.EntityFactory;
import org.symphonyoss.s2.canon.runtime.IBuilderFactory;
import org.symphonyoss.s2.common.type.provider.IBooleanProvider;
import org.symphonyoss.s2.common.type.provider.IStringProvider;
import org.symphonyoss.s2.common.type.provider.IIntegerProvider;
import org.symphonyoss.s2.common.type.provider.ILongProvider;
import org.symphonyoss.s2.common.type.provider.IFloatProvider;
import org.symphonyoss.s2.common.type.provider.IDoubleProvider;
import org.symphonyoss.s2.common.type.provider.IImmutableByteArrayProvider;
import org.symphonyoss.s2.common.dom.json.IJsonDomNode;
import org.symphonyoss.s2.common.dom.json.IImmutableJsonDomNode;
import org.symphonyoss.s2.common.dom.json.ImmutableJsonList;
import org.symphonyoss.s2.common.dom.json.ImmutableJsonSet;
import org.symphonyoss.s2.common.dom.json.ImmutableJsonObject;
import org.symphonyoss.s2.common.dom.json.MutableJsonList;
import org.symphonyoss.s2.common.dom.json.MutableJsonSet;
import org.symphonyoss.s2.common.dom.json.MutableJsonObject;
import org.symphonyoss.s2.common.dom.json.JsonArray;
import org.symphonyoss.s2.common.dom.json.JsonList;
import org.symphonyoss.s2.common.dom.json.JsonSet;
import java.util.HashSet;
import java.util.List;
import java.util.LinkedList;
import java.util.Set;
import java.util.Iterator;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableSet;
import java.util.List;
import java.util.LinkedList;
import com.google.common.collect.ImmutableList;
import org.symphonyoss.s2.common.immutable.ImmutableByteArray;
import com.symphony.oss.models.fundamental.canon.facade.*;
import com.symphony.oss.models.crypto.canon.facade.*;
import com.symphony.oss.models.crypto.canon.*;
import com.symphony.oss.models.core.canon.facade.*;
import com.symphony.oss.models.core.canon.*;
/**
* Object ObjectSchema(SecurityContextMember)
*
* wrappedKey is the SecurityContext SecretKey wrapped by the member's Public ExchangeKey. encryptedKey is the SecurityContext SecretKey encrypted with the member's AccountSecretKey. This may be added as an update by the member as a performance optimization.
* Generated from ObjectSchema(SecurityContextMember) at #/components/schemas/SecurityContextMember
*/
@Immutable
@SuppressWarnings("unused")
public abstract class SecurityContextMemberEntity extends Member
implements ISecurityContextMember, IFundamentalModelEntity
{
/** Type ID */
public static final String TYPE_ID = "com.symphony.s2.model.fundamental.SecurityContextMember";
/** Type version */
public static final String TYPE_VERSION = "1.0";
/** Type major version */
public static final Integer TYPE_MAJOR_VERSION = 1;
/** Type minor version */
public static final Integer TYPE_MINOR_VERSION = 0;
/** Factory instance */
public static final Factory FACTORY = new Factory();
/**
* Builder factory instance
*
* @deprecated use new SecurityContextMember.Builder()
or new SecurityContextMember.Builder(ISecurityContextMemberEntity)
*/
@Deprecated
public static final IBuilderFactory BUILDER = new BuilderFactory();
private final ImmutableSet unknownKeys_;
private final SecurityContextPermission _permission_;
/**
* Constructor from builder.
*
* @param builder A mutable builder containing all values.
*/
public SecurityContextMemberEntity(SecurityContextMember.AbstractSecurityContextMemberBuilder,?> builder)
{
super(builder);
_permission_ = builder.getPermission();
if(_permission_ == null)
throw new IllegalArgumentException("_permission_ is required.");
unknownKeys_ = ImmutableSet.of();
}
/**
* Set the _type attribute of the given mutable JSON object to the type ID of this type if it is null and
* return an immutable copy.
*
* @param mutableJsonObject A mutable JSON Object.
*
* @return An immutable copy of the given object with the _type attribute set.
*/
public static ImmutableJsonObject setType(MutableJsonObject mutableJsonObject)
{
if(mutableJsonObject.get(CanonRuntime.JSON_TYPE) == null)
mutableJsonObject.addIfNotNull(CanonRuntime.JSON_TYPE, TYPE_ID);
return mutableJsonObject.immutify();
}
/**
* Constructor from mutable JSON object.
*
* @param mutableJsonObject A mutable JSON object containing the serialized form of the object.
* @param modelRegistry A model registry to use to deserialize any nested objects.
*/
public SecurityContextMemberEntity(MutableJsonObject mutableJsonObject, IModelRegistry modelRegistry)
{
this(setType(mutableJsonObject), modelRegistry);
}
/**
* Constructor from serialised form.
*
* @param jsonObject An immutable JSON object containing the serialized form of the object.
* @param modelRegistry A model registry to use to deserialize any nested objects.
*/
public SecurityContextMemberEntity(ImmutableJsonObject jsonObject, IModelRegistry modelRegistry)
{
super(jsonObject, modelRegistry);
if(jsonObject == null)
throw new IllegalArgumentException("jsonObject is required");
Set keySet = new HashSet<>(super.getCanonUnknownKeys());
if(keySet.remove("permission"))
{
IJsonDomNode node = jsonObject.get("permission");
if(node instanceof IStringProvider)
{
String value = ((IStringProvider)node).asString();
try
{
_permission_ = SecurityContextPermission.valueOf(value);
}
catch(RuntimeException e)
{
throw new IllegalArgumentException("Value \"" + value + "\" for permission is not a valid value", e);
}
}
else
{
throw new IllegalArgumentException("permission must be an instance of String not " + node.getClass().getName());
}
}
else
{
throw new IllegalArgumentException("permission is required.");
}
unknownKeys_ = ImmutableSet.copyOf(keySet);
}
/**
* Copy constructor.
*
* @param other Another instance from which all attributes are to be copied.
*/
public SecurityContextMemberEntity(ISecurityContextMember other)
{
super(other);
_permission_ = other.getPermission();
unknownKeys_ = other.getCanonUnknownKeys();
}
@Override
public ImmutableSet getCanonUnknownKeys()
{
return unknownKeys_;
}
@Override
public SecurityContextPermission getPermission()
{
return _permission_;
}
@Override
public boolean equals(Object obj)
{
if(obj instanceof SecurityContextMemberEntity)
return toString().equals(((SecurityContextMemberEntity)obj).toString());
return false;
}
/**
* Factory class for SecurityContextMember.
*/
public static class Factory extends EntityFactory
{
protected Factory()
{
super(ISecurityContextMember.class, ISecurityContextMemberEntity.class);
}
/**
* Return the type identifier (_type JSON attribute) for entities created by this factory.
*
* @return The type identifier for entities created by this factory.
*/
@Override
public String getCanonType()
{
return TYPE_ID;
}
/**
* Return the type version (_version JSON attribute) for entities created by this factory.
*
* @return The type version for entities created by this factory.
*/
public String getCanonVersion()
{
return TYPE_VERSION;
}
/**
* Return the major type version for entities created by this factory.
*
* @return The major type version for entities created by this factory.
*/
public @Nullable Integer getCanonMajorVersion()
{
return TYPE_MAJOR_VERSION;
}
/**
* Return the minjor type version for entities created by this factory.
*
* @return The minor type version for entities created by this factory.
*/
public @Nullable Integer getCanonMinorVersion()
{
return TYPE_MINOR_VERSION;
}
/**
* Return a new entity instance created from the given JSON serialization.
*
* @param jsonObject The JSON serialized form of the required entity.
* @param modelRegistry A model registry to use to deserialize any nested objects.
*
* @return An instance of the entity represented by the given serialized form.
*
* @throws IllegalArgumentException If the given JSON is not valid.
*/
@Override
public ISecurityContextMember newInstance(ImmutableJsonObject jsonObject, IModelRegistry modelRegistry)
{
return new SecurityContextMember(jsonObject, modelRegistry);
}
/**
* Return a new entity instance created from the given builder instance.
* This is used to construct an entity from its builder as the builder also
* implements the interface of the entity.
*
* @param builder a builder containing values of all fields for the required entity.
*
* @return An instance of the entity represented by the given values.
*
* @throws IllegalArgumentException If the given values are not valid.
*/
public ISecurityContextMember newInstance(Builder builder)
{
return new SecurityContextMember(builder);
}
}
/**
* Builder factory
*
* @deprecated use new SecurityContextMember.Builder()
or new SecurityContextMember.Builder(ISecurityContextMemberEntity)
*/
@Deprecated
private static class BuilderFactory implements IBuilderFactory
{
/**
* @deprecated use new SecurityContextMember.Builder()
*/
@Deprecated
@Override
public Builder newInstance()
{
return new Builder();
}
/**
* @deprecated use new SecurityContextMember.Builder(ISecurityContextMemberEntity)
*/
@Deprecated
@Override
public Builder newInstance(ISecurityContextMemberEntity initial)
{
return new Builder(initial);
}
}
/**
* Builder for SecurityContextMember
*
* Created by calling BUILDER.newInstance();
*
*/
public static class Builder extends SecurityContextMember.AbstractSecurityContextMemberBuilder
{
/**
* Constructor.
*/
public Builder()
{
super(Builder.class);
}
/**
* Constructor initialised from another object instance.
*
* @param initial An instance of the built type from which values are to be initialised.
*/
public Builder(ISecurityContextMemberEntity initial)
{
super(Builder.class, initial);
}
@Override
protected ISecurityContextMember construct()
{
return new SecurityContextMember(this);
}
}
/**
* Abstract builder for SecurityContextMember. If there are sub-classes of this type then their builders sub-class this builder.
*
* @param The concrete type of the builder, used for fluent methods.
* @param The concrete type of the built object.
*/
public static abstract class AbstractSecurityContextMemberEntityBuilder, T extends ISecurityContextMemberEntity>
extends Member.AbstractMemberBuilder
{
protected SecurityContextPermission _permission_;
protected AbstractSecurityContextMemberEntityBuilder(Class type)
{
super(type);
}
protected AbstractSecurityContextMemberEntityBuilder(Class type, ISecurityContextMemberEntity initial)
{
super(type, initial);
_permission_ = initial.getPermission();
}
public B withValues(ImmutableJsonObject jsonObject, boolean ignoreValidation, IModelRegistry modelRegistry)
{
super.withValues(jsonObject, ignoreValidation, modelRegistry);
if(jsonObject.containsKey("permission"))
{
IJsonDomNode node = jsonObject.get("permission");
if(node instanceof IStringProvider)
{
String value = ((IStringProvider)node).asString();
try
{
_permission_ = SecurityContextPermission.valueOf(value);
}
catch(RuntimeException e)
{
if(!ignoreValidation) throw new IllegalArgumentException("Value \"" + value + "\" for permission is not a valid value", e);
}
}
else if(!ignoreValidation)
{
throw new IllegalArgumentException("permission must be an instance of String not " + node.getClass().getName());
}
}
return self();
}
public void populateAllFields(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy