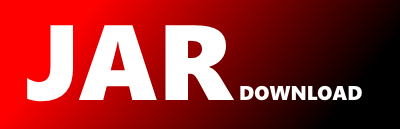
utils.DataProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symphony-api-client-java Show documentation
Show all versions of symphony-api-client-java Show documentation
Symphony API Client provided by Symphony Platform Solutions team
package utils;
import clients.SymBotClient;
import exceptions.SymClientException;
import model.UserInfo;
import org.symphonyoss.symphony.messageml.exceptions.InvalidInputException;
import org.symphonyoss.symphony.messageml.util.IDataProvider;
import org.symphonyoss.symphony.messageml.util.IUserPresentation;
import javax.ws.rs.core.NoContentException;
import java.net.URI;
import java.util.HashSet;
import java.util.Set;
public class DataProvider implements IDataProvider {
private static final Set STANDARD_URI_SCHEMES = new HashSet<>();
private UserPresentation user;
private SymBotClient botClient;
public DataProvider(SymBotClient botClient) {
STANDARD_URI_SCHEMES.add("http");
STANDARD_URI_SCHEMES.add("https");
this.botClient = botClient;
}
@Override
public IUserPresentation getUserPresentation(String email) throws InvalidInputException {
if (!email.equalsIgnoreCase(user.getEmail())) {
throw new InvalidInputException("Failed to lookup user \"" + email + "\"");
}
return new UserPresentation(user.getId(), user.getScreenName(), user.getPrettyName(), email);
}
@Override
public IUserPresentation getUserPresentation(Long uid) throws InvalidInputException {
UserInfo userInfo = null;
try {
userInfo = botClient.getUsersClient().getUserFromId(uid, false);
} catch (SymClientException e) {
e.printStackTrace();
} catch (NoContentException e) {
e.printStackTrace();
}
return new UserPresentation(uid, userInfo.getDisplayName(),userInfo.getDisplayName());
}
@Override
public void validateURI(URI uri) throws InvalidInputException {
if (!STANDARD_URI_SCHEMES.contains(uri.getScheme().toLowerCase())) {
throw new InvalidInputException(
"URI scheme \"" + uri.getScheme() + "\" is not supported by the pod.");
}
}
public void setUserPresentation(UserPresentation user) {
this.user = user;
}
public void setUserPresentation(long id, String screenName, String prettyName, String email) {
this.user = new UserPresentation(id, screenName, prettyName, email);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy