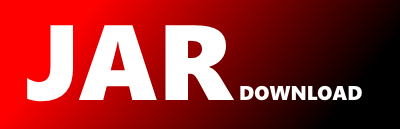
services.DatafeedEventsService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symphony-api-client-java Show documentation
Show all versions of symphony-api-client-java Show documentation
Symphony API Client provided by Symphony Platform Solutions team
package services;
import clients.SymBotClient;
import clients.symphony.api.DatafeedClient;
import configuration.SymConfig;
import configuration.SymLoadBalancedConfig;
import exceptions.SymClientException;
import listeners.ConnectionListener;
import listeners.DatafeedListener;
import listeners.IMListener;
import listeners.RoomListener;
import model.DatafeedEvent;
import model.events.MessageSent;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.ws.rs.ProcessingException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.*;
import java.util.concurrent.atomic.AtomicBoolean;
public class DatafeedEventsService {
private final Logger logger = LoggerFactory.getLogger(DatafeedEventsService.class);
private SymBotClient botClient;
private DatafeedClient datafeedClient;
private List roomListeners;
private List imListeners;
private List connectionListeners;
private String datafeedId = null;
private ExecutorService pool;
private AtomicBoolean stop = new AtomicBoolean();
private final int THREADPOOL_SIZE;
private final int TIMEOUT_NO_OF_SECS;
public DatafeedEventsService(SymBotClient client) {
this.botClient = client;
this.THREADPOOL_SIZE = client.getConfig().getDatafeedEventsThreadpoolSize() != 0
? client.getConfig().getDatafeedEventsThreadpoolSize() : 5;
this.TIMEOUT_NO_OF_SECS = client.getConfig().getDatafeedEventsErrorTimeout() != 0
? client.getConfig().getDatafeedEventsErrorTimeout() : 30;
roomListeners = new ArrayList<>();
imListeners = new ArrayList<>();
connectionListeners = new ArrayList<>();
datafeedClient = this.botClient.getDatafeedClient();
while (datafeedId == null) {
try {
datafeedId = datafeedClient.createDatafeed();
} catch (ProcessingException e) {
handleError(e);
}
}
readDatafeed();
stop.set(false);
}
public void addListeners(DatafeedListener... listeners) {
for (DatafeedListener listener : listeners) {
if (listener instanceof RoomListener) {
roomListeners.add((RoomListener) listener);
} else if (listener instanceof IMListener) {
imListeners.add((IMListener) listener);
} else if (listener instanceof ConnectionListener) {
connectionListeners.add((ConnectionListener) listener);
}
}
}
public void addRoomListener(RoomListener listener) {
roomListeners.add(listener);
}
public void removeRoomListener(RoomListener listener) {
roomListeners.remove(listener);
}
public void addIMListener(IMListener listener) {
imListeners.add(listener);
}
public void removeIMListener(IMListener listener) {
imListeners.remove(listener);
}
public void addConnectionsListener(ConnectionListener listener) {
connectionListeners.add(listener);
}
public void removeConnectionsListener(ConnectionListener listener) {
connectionListeners.remove(listener);
}
public void readDatafeed() {
if (pool != null) {
pool.shutdown();
}
pool = Executors.newFixedThreadPool(THREADPOOL_SIZE);
CompletableFuture.supplyAsync(() -> {
while (!stop.get()) {
CompletableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy