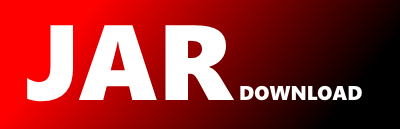
clients.symphony.api.InformationBarriersClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symphony-api-client-java Show documentation
Show all versions of symphony-api-client-java Show documentation
Symphony API Client provided by Symphony Platform Solutions team
package clients.symphony.api;
import clients.ISymClient;
import clients.symphony.api.constants.CommonConstants;
import clients.symphony.api.constants.PodConstants;
import exceptions.SymClientException;
import exceptions.UnauthorizedException;
import model.InformationBarrierGroup;
import model.InformationBarrierGroupStatus;
import model.Policy;
import javax.ws.rs.client.Entity;
import javax.ws.rs.client.Invocation;
import javax.ws.rs.core.GenericType;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import java.util.List;
public class InformationBarriersClient extends APIClient {
private ISymClient botClient;
private static String podTarget;
public InformationBarriersClient(ISymClient client) {
botClient = client;
podTarget = CommonConstants.HTTPS_PREFIX + botClient.getConfig().getPodHost();
if (botClient.getConfig().getPodPort() != 443) {
podTarget += ":" + botClient.getConfig().getPodPort();
}
}
public List listGroups() throws SymClientException {
Invocation.Builder builder = botClient.getPodClient()
.target(podTarget)
.path(PodConstants.LISTIBGROUPS)
.request(MediaType.APPLICATION_JSON)
.header("sessionToken", botClient.getSymAuth().getSessionToken());
try (Response response = builder.get()) {
if (response.getStatusInfo().getFamily() != Response.Status.Family.SUCCESSFUL) {
try {
handleError(response, botClient);
} catch (UnauthorizedException ex) {
return listGroups();
}
return null;
} else {
return response.readEntity(new GenericType>() {});
}
}
}
public List listGroupMembers(String groupId) throws SymClientException {
Invocation.Builder builder = botClient.getPodClient()
.target(podTarget)
.path(PodConstants.LISTIBGROUPMEMBERS.replace("{gid}", groupId))
.request(MediaType.APPLICATION_JSON)
.header("sessionToken", botClient.getSymAuth().getSessionToken());
try (Response response = builder.get()) {
if (response.getStatusInfo().getFamily() != Response.Status.Family.SUCCESSFUL) {
try {
handleError(response, botClient);
} catch (UnauthorizedException ex) {
return listGroupMembers(groupId);
}
return null;
} else {
return response.readEntity(new GenericType>() {});
}
}
}
public InformationBarrierGroupStatus addGroupMembers(String groupId, List members) throws SymClientException {
Invocation.Builder builder = botClient.getPodClient()
.target(podTarget)
.path(PodConstants.ADDIBGROUPMEMBERS.replace("{gid}", groupId))
.request(MediaType.APPLICATION_JSON)
.header("sessionToken", botClient.getSymAuth().getSessionToken());
try (Response response = builder.post(Entity.entity(members, MediaType.APPLICATION_JSON))) {
if (response.getStatusInfo().getFamily() != Response.Status.Family.SUCCESSFUL) {
try {
handleError(response, botClient);
} catch (UnauthorizedException ex) {
return addGroupMembers(groupId, members);
}
return null;
} else {
return response.readEntity(InformationBarrierGroupStatus.class);
}
}
}
public InformationBarrierGroupStatus removeGroupMembers(String groupId, List members) throws SymClientException {
Invocation.Builder builder = botClient.getPodClient()
.target(podTarget)
.path(PodConstants.REMOVEIBGROUPMEMBERS.replace("{gid}", groupId))
.request(MediaType.APPLICATION_JSON)
.header("sessionToken", botClient.getSymAuth().getSessionToken());
try (Response response = builder.post(Entity.entity(members, MediaType.APPLICATION_JSON))) {
if (response.getStatusInfo().getFamily() != Response.Status.Family.SUCCESSFUL) {
try {
handleError(response, botClient);
} catch (UnauthorizedException ex) {
return removeGroupMembers(groupId, members);
}
return null;
} else {
return response.readEntity(InformationBarrierGroupStatus.class);
}
}
}
public List listPolicies() throws SymClientException {
Invocation.Builder builder = botClient.getPodClient()
.target(podTarget)
.path(PodConstants.LISTPOLICIES)
.request(MediaType.APPLICATION_JSON)
.header("sessionToken", botClient.getSymAuth().getSessionToken());
try (Response response = builder.get()) {
if (response.getStatusInfo().getFamily() != Response.Status.Family.SUCCESSFUL) {
try {
handleError(response, botClient);
} catch (UnauthorizedException ex) {
return listPolicies();
}
return null;
} else {
return response.readEntity(new GenericType>() {});
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy