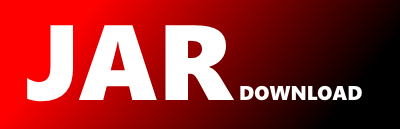
utils.MessageUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symphony-api-client-java Show documentation
Show all versions of symphony-api-client-java Show documentation
Symphony API Client provided by Symphony Platform Solutions team
package utils;
import java.util.LinkedHashMap;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.lang3.StringUtils;
public class MessageUtils {
private static LinkedHashMap tokens = null;
private static Pattern pattern = null;
private static void init() {
if (tokens != null) {
return;
}
tokens = new LinkedHashMap<>();
tokens.put("&", "&");
tokens.put("<", "<");
tokens.put(">", ">");
tokens.put("'", "'");
tokens.put("\"", """);
tokens.put("\\$", "$");
tokens.put("#", "#");
tokens.put("\\(", "(");
tokens.put("\\)", ")");
tokens.put("=", "=");
tokens.put(";", ";");
tokens.put("\\\\", "\");
tokens.put("\\.", ".");
tokens.put("`", "`");
tokens.put("%", "%");
tokens.put("\\*", "*");
tokens.put("\\[", "[");
tokens.put("\\]", "]");
tokens.put("\\{", "{");
tokens.put("\\}", "}");
pattern = Pattern.compile("(" + StringUtils.join(tokens.keySet(), "|") + ")");
}
public static String escapeText(String rawText) {
init();
Matcher matcher = pattern.matcher(rawText);
StringBuffer buffer = new StringBuffer();
while (matcher.find()) {
String group = matcher.group(1);
String replacement = tokens.get(group);
if (replacement == null) {
replacement = tokens.get("\\" + group);
}
matcher.appendReplacement(buffer, replacement);
}
matcher.appendTail(buffer);
return buffer.toString();
}
public static String escapeStreamId(String rawStreamId) {
return rawStreamId.trim()
.replaceAll("[=]+$", "")
.replaceAll("\\+", "-")
.replaceAll("/", "_");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy