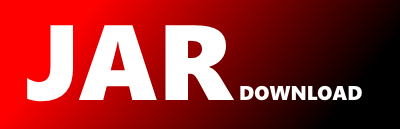
com.syncthemall.diffbot.model.frontpage.Frontpage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of diffbot-java-sdk Show documentation
Show all versions of diffbot-java-sdk Show documentation
API for http://www.diffbot.com/ services.
/**
* Copyright (c) 2013 Pierre-Denis Vanduynslager, https://github.com/vanduynslagerp
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the
* "Software"), to deal in the Software without restriction, including
* without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to
* permit persons to whom the Software is furnished to do so, subject to
* the following conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.syncthemall.diffbot.model.frontpage;
import java.io.Serializable;
import java.util.List;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* The result of a front page extraction by Diffbot (Frontpage API).
*/
@XmlRootElement(name = "dml")
public final class Frontpage implements Serializable {
/** Serial code version serialVersionUID
. **/
private static final long serialVersionUID = 7531133216091403402L;
private long id;
private List- items;
private Info info;
/**
* Default constructor.
*/
public Frontpage() {
super();
}
@Override
public String toString() {
return String.format("Frontpage [title=%s]", info.getTitle());
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((info.getSourceURL() == null) ? 0 : info.getSourceURL().hashCode());
return result;
}
@Override
public boolean equals(final Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Frontpage)) {
return false;
}
Frontpage other = (Frontpage) obj;
if (info.getSourceURL() == null) {
if (other.info.getSourceURL() != null) {
return false;
}
} else if (!info.getSourceURL().equals(other.info.getSourceURL())) {
return false;
}
return true;
}
/**
* @return the list of {@code Item} of the extracted page
*/
@XmlElement(name = "item")
public List
- getItems() {
return items;
}
/**
* @return the {@code Info} of the extracted page
*/
@XmlElement
public Info getInfo() {
return info;
}
/**
* @return the id of the extracted page (generated by Diffbot)
*/
@XmlElement
public long getId() {
return id;
}
/**
* @param items the list of {@code Item} of the extracted page
*/
public void setItems(final List
- items) {
this.items = items;
}
/**
* @param id the id of the extracted page (generated by Diffbot)
*/
public void setId(final long id) {
this.id = id;
}
/**
* @param info the {@code Info} of the extracted page
*/
public void setInfo(final Info info) {
this.info = info;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy