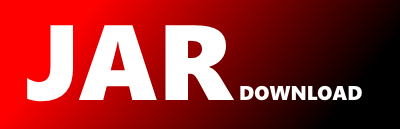
com.dropbox.client2.jsonextract.JsonMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dropbox-java-sdk Show documentation
Show all versions of dropbox-java-sdk Show documentation
The Dropbox API for Java is a Dropbox supported client library for accessing the
JSON+REST interface to Dropbox. It supports OAuth proxied authentication. It is
designed to be simple and easy to use, as well as instructional in case you want
to write your own. It is MIT licensed.
The newest version!
package com.dropbox.client2.jsonextract;
import java.util.Iterator;
import java.util.Map;
/**
* (Internal class for extracting JSON.)
*
* A JSON "object" (a mapping of string keys to arbitrary JSON values).
*/
public final class JsonMap extends JsonBase
© 2015 - 2024 Weber Informatics LLC | Privacy Policy