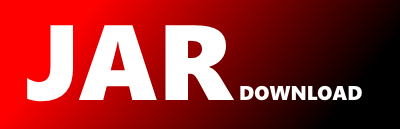
com.synedge.oss.client.invalidation.JerseyInvalidationClient.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of synedge-java-client Show documentation
Show all versions of synedge-java-client Show documentation
This client allows you to easily connect to the Synedge API with any language running on the JDK
The newest version!
package com.synedge.oss.client.invalidation
import com.synedge.oss.client.InvalidationClient
import com.synedge.oss.client.SynedgeClient
import com.synedge.oss.client.authentication.AuthenticatedClient
import com.synedge.oss.client.exceptions.SynedgeClientException
import com.synedge.oss.client.exceptions.SynedgeClientInvalidationException
import groovy.json.JsonOutput
import groovy.transform.CompileStatic
import groovy.transform.PackageScope
import javax.ws.rs.client.Entity
class JerseyInvalidationClient extends AuthenticatedClient implements InvalidationClient {
@PackageScope
JerseyInvalidationClient(String username, String password, String endpoint = SynedgeClient.DEFAULT_ENDPOINT) {
super(username, password, endpoint)
}
/**
* {@inheritDoc}
*
* @see com.synedge.oss.client.InvalidationClient#invalidate(java.lang.String, java.util.List)
*/
@CompileStatic
@Override
String invalidate(String customerId, Iterable urls) throws SynedgeClientInvalidationException {
for (String urlStr : urls) {
try {
URL url = new URL(urlStr)
if (!(url.protocol == 'http' || url.protocol == 'https')) {
throw new SynedgeClientInvalidationException('URL ' + url + ' is invalid, only http or https protocols are allowed')
}
if (!url.host?.trim()) {
throw new SynedgeClientInvalidationException('URL ' + url + ' is invalid, domain missing')
}
}
catch (MalformedURLException e) {
throw new SynedgeClientInvalidationException('URL ' + urlStr + ' is invalid', e)
}
}
String json = '{"list": ' + JsonOutput.toJson(urls) + '}'
try {
return signAndPost(client.target(endpoint).path(customerId).path('invalidate').request(), Entity.json(json))
}
catch (SynedgeClientException e) {
throw new SynedgeClientInvalidationException(e.message, e)
}
}
@Override
Invalidations getInvalidations(String customerId, int start, int count) {
def result = signAndGet(client.target(endpoint).path(customerId).path('invalidate').queryParam('start', start).queryParam('count', count).request())
new Invalidations(result.start, result.count,
result.status.collect { stat -> convertInvalidation(stat) }
)
}
@Override
Invalidation getInvalidation(String customerId, String invalidationId) {
convertInvalidation(signAndGet(client.target(endpoint).path(customerId).path('invalidate').path(invalidationId).request()))
}
private def convertInvalidation(def stat) {
new Invalidation(stat.invalidationId, InvalidationStatus.valueOf(stat.status),
stat.urlStatus.collect { urlStatus ->
new InvalidationUrl(urlStatus.url, urlStatus.distrubutionGroupExternalId, InvalidationStatus.valueOf(urlStatus.status), urlStatus.message)
}, new Date(stat.submitted), new Date(stat.completed))
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy