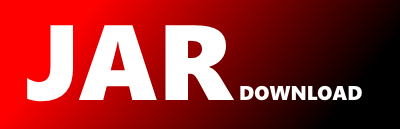
com.synerset.unitility.quarkus.serialization.PhysicalQuantityParamJakartaConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unitility-quarkus Show documentation
Show all versions of unitility-quarkus Show documentation
The Java Physics Units of Measure Library - Unitility, Quarkus extension.
This module integrates unitility-core and unitility-jackson. It provides a preconfigured Jackson module
and JakartaParamProvider, enabling automatic serialization/deserialization for all supported physical quantity
types. This configuration is automatically included through the Jandex index.
package com.synerset.unitility.quarkus.serialization;
import com.synerset.unitility.unitsystem.PhysicalQuantity;
import com.synerset.unitility.unitsystem.Unit;
import com.synerset.unitility.unitsystem.util.PhysicalQuantityParsingFactory;
import jakarta.ws.rs.ext.ParamConverter;
/**
* The PhysicalQuantityParamJakartaConverter class is a Jakarta RS {@link ParamConverter} for converting string
* representations to {@link PhysicalQuantity} instances and vice versa.
*
* @param The type of unit associated with the {@link PhysicalQuantity}.
* @param The type of {@link PhysicalQuantity}.
*/
public class PhysicalQuantityParamJakartaConverter> implements ParamConverter {
private final Class targetClass;
private final PhysicalQuantityParsingFactory parsingRegistry;
public PhysicalQuantityParamJakartaConverter(Class targetClass, PhysicalQuantityParsingFactory parsingRegistry) {
this.targetClass = targetClass;
this.parsingRegistry = parsingRegistry;
}
/**
* Converts a string representation to a {@link PhysicalQuantity} instance.
* This method is called by Jakarta RS to convert a string representation to a {@link PhysicalQuantity} instance
* using the {@link PhysicalQuantityParsingFactory}.
*
* @param quantityAsString The string representation of the {@link PhysicalQuantity}.
* @return A {@link PhysicalQuantity} instance.
*/
@Override
public Q fromString(String quantityAsString) {
return parsingRegistry.parse(targetClass, quantityAsString);
}
/**
* Converts a {@link PhysicalQuantity} instance to its string representation.
* Jakarta calls this method RS to convert a {@link PhysicalQuantity} instance to its string representation
* using the toEngineeringFormat() method.
*
* @param physicalQuantity The {@link PhysicalQuantity} instance to be converted.
* @return The string representation of the {@link PhysicalQuantity}.
*/
@Override
public String toString(Q physicalQuantity) {
return physicalQuantity.toEngineeringFormat();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy