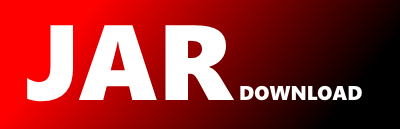
com.synopsys.integration.blackduck.api.generated.view.VulnerabilityViewV4 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blackduck-common-api Show documentation
Show all versions of blackduck-common-api Show documentation
A library of mostly temporary request/response classes for the Black Duck REST API.
/**
* blackduck-common-api
*
* Copyright (c) 2020 Synopsys, Inc.
*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.synopsys.integration.blackduck.api.generated.view;
import java.math.BigDecimal;
import java.util.List;
import java.util.Optional;
import com.synopsys.integration.blackduck.api.core.BlackDuckComponent;
import com.synopsys.integration.blackduck.api.core.BlackDuckView;
import com.synopsys.integration.blackduck.api.generated.component.VulnerabilityCvss2View;
import com.synopsys.integration.blackduck.api.generated.component.VulnerabilityCvss3View;
import com.synopsys.integration.blackduck.api.generated.enumeration.ProjectVersionVulnerableBomComponentsItemsVulnerabilityWithRemediationSeverityType;
import com.synopsys.integration.blackduck.api.generated.enumeration.ProjectVersionVulnerableBomComponentsItemsVulnerabilityWithRemediationSourceType;
/**
* this file should not be edited - if changes are necessary, the generator should be updated, then this file should be re-created
* **/
public class VulnerabilityViewV4 extends BlackDuckView {
private java.util.Date exploitPublishDate;
private ProjectVersionVulnerableBomComponentsItemsVulnerabilityWithRemediationSeverityType severity;
private String name;
private String solution;
private String title;
private Boolean useCvss3;
private java.util.Date disclosureDate;
private java.util.Date updatedDate;
private java.util.Date solutionDate;
private String technicalDescription;
private VulnerabilityCvss2View cvss2;
private java.util.List classifications;
private BigDecimal overallScore;
private ProjectVersionVulnerableBomComponentsItemsVulnerabilityWithRemediationSourceType source;
private String description;
private VulnerabilityCvss3View cvss3;
private java.util.Date publishedDate;
public java.util.Date getExploitPublishDate() {
return exploitPublishDate;
}
public void setExploitPublishDate(java.util.Date exploitPublishDate) {
this.exploitPublishDate = exploitPublishDate;
}
public ProjectVersionVulnerableBomComponentsItemsVulnerabilityWithRemediationSeverityType getSeverity() {
return severity;
}
public void setSeverity(ProjectVersionVulnerableBomComponentsItemsVulnerabilityWithRemediationSeverityType severity) {
this.severity = severity;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSolution() {
return solution;
}
public void setSolution(String solution) {
this.solution = solution;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public Boolean getUseCvss3() {
return useCvss3;
}
public void setUseCvss3(Boolean useCvss3) {
this.useCvss3 = useCvss3;
}
public java.util.Date getDisclosureDate() {
return disclosureDate;
}
public void setDisclosureDate(java.util.Date disclosureDate) {
this.disclosureDate = disclosureDate;
}
public java.util.Date getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(java.util.Date updatedDate) {
this.updatedDate = updatedDate;
}
public java.util.Date getSolutionDate() {
return solutionDate;
}
public void setSolutionDate(java.util.Date solutionDate) {
this.solutionDate = solutionDate;
}
public String getTechnicalDescription() {
return technicalDescription;
}
public void setTechnicalDescription(String technicalDescription) {
this.technicalDescription = technicalDescription;
}
public VulnerabilityCvss2View getCvss2() {
return cvss2;
}
public void setCvss2(VulnerabilityCvss2View cvss2) {
this.cvss2 = cvss2;
}
public java.util.List getClassifications() {
return classifications;
}
public void setClassifications(java.util.List classifications) {
this.classifications = classifications;
}
public BigDecimal getOverallScore() {
return overallScore;
}
public void setOverallScore(BigDecimal overallScore) {
this.overallScore = overallScore;
}
public ProjectVersionVulnerableBomComponentsItemsVulnerabilityWithRemediationSourceType getSource() {
return source;
}
public void setSource(ProjectVersionVulnerableBomComponentsItemsVulnerabilityWithRemediationSourceType source) {
this.source = source;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public VulnerabilityCvss3View getCvss3() {
return cvss3;
}
public void setCvss3(VulnerabilityCvss3View cvss3) {
this.cvss3 = cvss3;
}
public java.util.Date getPublishedDate() {
return publishedDate;
}
public void setPublishedDate(java.util.Date publishedDate) {
this.publishedDate = publishedDate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy