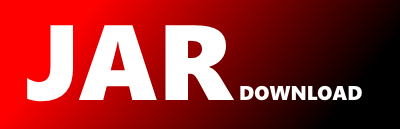
com.synopsys.integration.blackduck.service.dataservice.ProjectUsersService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blackduck-common Show documentation
Show all versions of blackduck-common Show documentation
A library for using various capabilities of Black Duck, notably the REST API and signature scanning.
/*
* blackduck-common
*
* Copyright (c) 2021 Synopsys, Inc.
*
* Use subject to the terms and conditions of the Synopsys End User Software License and Maintenance Agreement. All rights reserved worldwide.
*/
package com.synopsys.integration.blackduck.service.dataservice;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import com.synopsys.integration.blackduck.api.core.response.UrlMultipleResponses;
import com.synopsys.integration.blackduck.api.generated.discovery.ApiDiscovery;
import com.synopsys.integration.blackduck.api.generated.view.ProjectView;
import com.synopsys.integration.blackduck.api.generated.view.UserGroupView;
import com.synopsys.integration.blackduck.api.generated.view.UserView;
import com.synopsys.integration.blackduck.api.manual.temporary.component.AssignedUserGroupRequest;
import com.synopsys.integration.blackduck.api.manual.temporary.component.AssignedUserRequest;
import com.synopsys.integration.blackduck.api.manual.temporary.response.AssignedUserGroupView;
import com.synopsys.integration.blackduck.api.manual.temporary.view.AssignedUserView;
import com.synopsys.integration.blackduck.exception.BlackDuckIntegrationException;
import com.synopsys.integration.blackduck.service.BlackDuckApiClient;
import com.synopsys.integration.blackduck.service.DataService;
import com.synopsys.integration.exception.IntegrationException;
import com.synopsys.integration.log.IntLogger;
import com.synopsys.integration.rest.HttpUrl;
public class ProjectUsersService extends DataService {
private final UserGroupService userGroupService;
public ProjectUsersService(BlackDuckApiClient blackDuckApiClient, ApiDiscovery apiDiscovery, IntLogger logger, UserGroupService userGroupService) {
super(blackDuckApiClient, apiDiscovery, logger);
this.userGroupService = userGroupService;
}
public List getAssignedUsersToProject(ProjectView project) throws IntegrationException {
List assignedUsers = blackDuckApiClient.getAllResponses(project.metaUsersLink());
return assignedUsers;
}
public List getUsersForProject(ProjectView project) throws IntegrationException {
logger.debug("Attempting to get the assigned users for Project: " + project.getName());
List assignedUsers = getAssignedUsersToProject(project);
List resolvedUserViews = new ArrayList<>();
for (AssignedUserView assigned : assignedUsers) {
HttpUrl userUrl = new HttpUrl(assigned.getUser());
UserView userView = blackDuckApiClient.getResponse(userUrl, UserView.class);
if (userView != null) {
resolvedUserViews.add(userView);
}
}
return resolvedUserViews;
}
public List getAssignedGroupsToProject(ProjectView project) throws IntegrationException {
List assignedGroups = blackDuckApiClient.getAllResponses(project.metaUsergroupsLink());
return assignedGroups;
}
public List getGroupsForProject(ProjectView project) throws IntegrationException {
logger.debug("Attempting to get the assigned users for Project: " + project.getName());
List assignedGroups = getAssignedGroupsToProject(project);
List resolvedGroupViews = new ArrayList<>();
for (AssignedUserGroupView assigned : assignedGroups) {
HttpUrl groupUrl = new HttpUrl(assigned.getGroup());
UserGroupView groupView = blackDuckApiClient.getResponse(groupUrl, UserGroupView.class);
if (groupView != null) {
resolvedGroupViews.add(groupView);
}
}
return resolvedGroupViews;
}
/**
* This will get all explicitly assigned users for a project, as well as all users who are assigned to groups that are explicitly assigned to a project.
*/
public Set getAllActiveUsersForProject(ProjectView projectView) throws IntegrationException {
Set users = new HashSet<>();
List assignedGroups = getAssignedGroupsToProject(projectView);
for (AssignedUserGroupView assignedUserGroupView : assignedGroups) {
if (assignedUserGroupView.getActive()) {
HttpUrl groupUrl = new HttpUrl(assignedUserGroupView.getGroup());
UserGroupView userGroupView = blackDuckApiClient.getResponse(groupUrl, UserGroupView.class);
if (userGroupView.getActive()) {
List groupUsers = blackDuckApiClient.getAllResponses(userGroupView.metaUsersLink());
users.addAll(groupUsers);
}
}
}
List assignedUsers = getAssignedUsersToProject(projectView);
for (AssignedUserView assignedUser : assignedUsers) {
HttpUrl userUrl = new HttpUrl(assignedUser.getUser());
UserView userView = blackDuckApiClient.getResponse(userUrl, UserView.class);
users.add(userView);
}
return users
.stream()
.filter(UserView::getActive)
.collect(Collectors.toSet());
}
public void addGroupToProject(ProjectView projectView, String groupName) throws IntegrationException {
Optional optionalUserGroupView = userGroupService.getGroupByName(groupName);
UserGroupView userGroupView = optionalUserGroupView.orElseThrow(() -> new IntegrationException(String.format("The supplied group name (%s) does not exist.", groupName)));
HttpUrl userGroupUrl = userGroupView.getHref();
HttpUrl createUrl = projectView.getFirstLink(ProjectView.USERGROUPS_LINK);
AssignedUserGroupRequest userGroupRequest = new AssignedUserGroupRequest();
userGroupRequest.setGroup(userGroupUrl.string());
blackDuckApiClient.post(createUrl, userGroupRequest);
}
public void addUserToProject(ProjectView projectView, String username) throws IntegrationException {
UrlMultipleResponses userResponses = apiDiscovery.metaUsersLink();
List allUsers = blackDuckApiClient.getAllResponses(userResponses);
UserView userView = null;
for (UserView user : allUsers) {
if (user.getUserName().equalsIgnoreCase(username)) {
userView = user;
}
}
if (null == userView) {
throw new BlackDuckIntegrationException(String.format("The user (%s) does not exist.", username));
}
addUserToProject(projectView, userView);
}
public void addUserToProject(ProjectView projectView, UserView userView) throws IntegrationException {
AssignedUserRequest assignedUserRequest = new AssignedUserRequest();
HttpUrl userUrl = userView.getHref();
assignedUserRequest.setUser(userUrl.string());
HttpUrl addUserUrl = projectView.getFirstLink(ProjectView.USERS_LINK);
blackDuckApiClient.post(addUserUrl, assignedUserRequest);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy