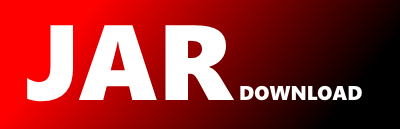
com.synopsys.integration.blackduck.service.dataservice.NotificationService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blackduck-common Show documentation
Show all versions of blackduck-common Show documentation
A library for using various capabilities of Black Duck, notably the REST API and signature scanning.
/*
* blackduck-common
*
* Copyright (c) 2023 Synopsys, Inc.
*
* Use subject to the terms and conditions of the Synopsys End User Software License and Maintenance Agreement. All rights reserved worldwide.
*/
package com.synopsys.integration.blackduck.service.dataservice;
import java.util.Date;
import java.util.List;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import com.synopsys.integration.blackduck.api.core.response.UrlMultipleResponses;
import com.synopsys.integration.blackduck.api.generated.discovery.ApiDiscovery;
import com.synopsys.integration.blackduck.api.generated.view.UserView;
import com.synopsys.integration.blackduck.api.manual.temporary.enumeration.NotificationType;
import com.synopsys.integration.blackduck.api.manual.view.NotificationUserView;
import com.synopsys.integration.blackduck.api.manual.view.NotificationView;
import com.synopsys.integration.blackduck.http.BlackDuckPageDefinition;
import com.synopsys.integration.blackduck.http.BlackDuckPageResponse;
import com.synopsys.integration.blackduck.http.BlackDuckRequestBuilder;
import com.synopsys.integration.blackduck.http.BlackDuckRequestFilter;
import com.synopsys.integration.blackduck.service.BlackDuckApiClient;
import com.synopsys.integration.blackduck.service.DataService;
import com.synopsys.integration.blackduck.service.request.BlackDuckMultipleRequest;
import com.synopsys.integration.blackduck.service.request.NotificationEditor;
import com.synopsys.integration.exception.IntegrationException;
import com.synopsys.integration.log.IntLogger;
public class NotificationService extends DataService {
// ejk - to get all notifications:
// /api/notifications?startDate=2019-07-01T00:00:00.000Z&endDate=2019-07-15T00:00:00.000Z&filter=notificationType:BOM_EDIT&filter=notificationType:LICENSE_LIMIT&filter=notificationType:POLICY_OVERRIDE&filter=notificationType:RULE_VIOLATION&filter=notificationType:RULE_VIOLATION_CLEARED&filter=notificationType:VERSION_BOM_CODE_LOCATION_BOM_COMPUTED&filter=notificationType:VULNERABILITY&filter=notificationType:PROJECT&filter=notificationType:PROJECT_VERSION
private final UrlMultipleResponses notificationsResponses = apiDiscovery.metaMultipleResponses(ApiDiscovery.NOTIFICATIONS_PATH);
private final Function> userNotificationsResponses = (userView) -> userView.metaNotificationsLink();
public NotificationService(BlackDuckApiClient blackDuckApiClient, ApiDiscovery apiDiscovery, IntLogger logger) {
super(blackDuckApiClient, apiDiscovery, logger);
}
public List getAllNotifications(NotificationEditor notificationEditor) throws IntegrationException {
BlackDuckRequestBuilder blackDuckRequestBuilder = createNotificationRequestBuilder(notificationEditor);
BlackDuckMultipleRequest requestMultiple = blackDuckRequestBuilder.buildBlackDuckRequest(notificationsResponses);
List possiblyPoorlyFilteredNotifications = blackDuckApiClient.getAllResponses(requestMultiple);
return reallyFilterNotifications(possiblyPoorlyFilteredNotifications, notificationEditor.getNotificationTypesToInclude());
}
public List getAllUserNotifications(UserView userView, NotificationEditor notificationEditor) throws IntegrationException {
BlackDuckRequestBuilder blackDuckRequestBuilder = createNotificationRequestBuilder(notificationEditor);
BlackDuckMultipleRequest requestMultiple = blackDuckRequestBuilder.buildBlackDuckRequest(userNotificationsResponses.apply(userView));
List possiblyPoorlyFilteredNotifications = blackDuckApiClient.getAllResponses(requestMultiple);
return reallyFilterNotifications(possiblyPoorlyFilteredNotifications, notificationEditor.getNotificationTypesToInclude());
}
public BlackDuckPageResponse getPageOfNotifications(NotificationEditor notificationEditor, BlackDuckPageDefinition blackDuckPageDefinition) throws IntegrationException {
BlackDuckRequestBuilder blackDuckRequestBuilder = createNotificationRequestBuilder(notificationEditor)
.setBlackDuckPageDefinition(blackDuckPageDefinition);
BlackDuckMultipleRequest requestMultiple = blackDuckRequestBuilder.buildBlackDuckRequest(notificationsResponses);
return blackDuckApiClient.getPageResponse(requestMultiple);
}
public BlackDuckPageResponse getPageOfUserNotifications(UserView userView, NotificationEditor notificationEditor, BlackDuckPageDefinition blackDuckPageDefinition) throws IntegrationException {
BlackDuckRequestBuilder blackDuckRequestBuilder = createNotificationRequestBuilder(notificationEditor)
.setBlackDuckPageDefinition(blackDuckPageDefinition);
BlackDuckMultipleRequest requestMultiple = blackDuckRequestBuilder.buildBlackDuckRequest(userNotificationsResponses.apply(userView));
return blackDuckApiClient.getPageResponse(requestMultiple);
}
/**
* @return The java.util.Date of the most recent notification. If there are no notifications, the current date will be returned. This can set an initial start time window for all future notifications.
* @throws IntegrationException
*/
public Date getLatestNotificationDate() throws IntegrationException {
BlackDuckRequestBuilder blackDuckRequestBuilder = createLatestDateRequestBuilder();
BlackDuckMultipleRequest requestMultiple = blackDuckRequestBuilder.buildBlackDuckRequest(notificationsResponses);
List notifications = blackDuckApiClient.getSomeResponses(requestMultiple, 1);
return getFirstCreatedAtDate(notifications);
}
/**
* @return The java.util.Date of the most recent notification in the user's stream. If there are no notifications, the current date will be returned. This can set an initial start time window for all future notifications.
* @throws IntegrationException
*/
public Date getLatestUserNotificationDate(UserView userView) throws IntegrationException {
BlackDuckRequestBuilder blackDuckRequestBuilder = createLatestDateRequestBuilder();
BlackDuckMultipleRequest requestMultiple = blackDuckRequestBuilder.buildBlackDuckRequest(userNotificationsResponses.apply(userView));
List userNotifications = blackDuckApiClient.getSomeResponses(requestMultiple, 1);
return getFirstCreatedAtDate(userNotifications);
}
private Date getFirstCreatedAtDate(List extends NotificationView> notifications) {
if (notifications.size() == 1) {
return notifications.get(0).getCreatedAt();
} else {
return new Date();
}
}
private BlackDuckRequestBuilder createLatestDateRequestBuilder() {
return new BlackDuckRequestBuilder()
.commonGet()
.addBlackDuckFilter(createFilterForAllKnownTypes());
}
private List getAllKnownNotificationTypes() {
List allKnownTypes = Stream.of(NotificationType.values()).map(Enum::name).collect(Collectors.toList());
return allKnownTypes;
}
private BlackDuckRequestFilter createFilterForAllKnownTypes() {
return createFilterForSpecificTypes(getAllKnownNotificationTypes());
}
private BlackDuckRequestFilter createFilterForSpecificTypes(List notificationTypesToInclude) {
return BlackDuckRequestFilter.createFilterWithMultipleValues("notificationType", notificationTypesToInclude);
}
private BlackDuckRequestBuilder createNotificationRequestBuilder(NotificationEditor notificationEditor) {
return new BlackDuckRequestBuilder()
.commonGet()
.apply(notificationEditor);
}
/*
ejk - We can not trust the filtering from the Black Duck API. There have
been at least 2 instances where the lack of filtering created customer
issues, so we will do this in perpetuity.
*/
private List reallyFilterNotifications(List notifications, List notificationTypesToInclude) {
return notifications
.stream()
.filter(notification -> notificationTypesToInclude.contains(notification.getType().name()))
.collect(Collectors.toList());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy