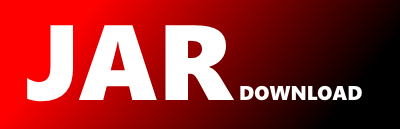
com.synopsys.integration.coverity.ws.v9.ConfigurationService Maven / Gradle / Ivy
/**
* coverity-common
*
* Copyright (C) 2018 Black Duck Software, Inc.
* http://www.blackducksoftware.com/
*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.synopsys.integration.coverity.ws.v9;
import java.util.List;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebResult;
import javax.jws.WebService;
import javax.xml.datatype.XMLGregorianCalendar;
import javax.xml.ws.RequestWrapper;
import javax.xml.ws.ResponseWrapper;
/**
* This class was generated by the JAX-WS RI.
* JAX-WS RI 2.2.4-b01
* Generated source version: 2.0
*/
@WebService(name = "ConfigurationService", targetNamespace = "http://ws.coverity.com/v9")
public interface ConfigurationService {
/**
* @param attributeDefinitionId
* @param attributeDefinitionSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateAttribute", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateAttribute")
@ResponseWrapper(localName = "updateAttributeResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateAttributeResponse")
public void updateAttribute(
@WebParam(name = "attributeDefinitionId", targetNamespace = "")
AttributeDefinitionIdDataObj attributeDefinitionId,
@WebParam(name = "attributeDefinitionSpec", targetNamespace = "")
AttributeDefinitionSpecDataObj attributeDefinitionSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param attributeDefinitionId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteAttribute", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteAttribute")
@ResponseWrapper(localName = "deleteAttributeResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteAttributeResponse")
public void deleteAttribute(
@WebParam(name = "attributeDefinitionId", targetNamespace = "")
AttributeDefinitionIdDataObj attributeDefinitionId)
throws CovRemoteServiceException_Exception
;
/**
* @param componentMapSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createComponentMap", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateComponentMap")
@ResponseWrapper(localName = "createComponentMapResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateComponentMapResponse")
public void createComponentMap(
@WebParam(name = "componentMapSpec", targetNamespace = "")
ComponentMapSpecDataObj componentMapSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param componentMapSpec
* @param componentMapId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateComponentMap", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateComponentMap")
@ResponseWrapper(localName = "updateComponentMapResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateComponentMapResponse")
public void updateComponentMap(
@WebParam(name = "componentMapId", targetNamespace = "")
ComponentMapIdDataObj componentMapId,
@WebParam(name = "componentMapSpec", targetNamespace = "")
ComponentMapSpecDataObj componentMapSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param componentMapId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteComponentMap", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteComponentMap")
@ResponseWrapper(localName = "deleteComponentMapResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteComponentMapResponse")
public void deleteComponentMap(
@WebParam(name = "componentMapId", targetNamespace = "")
ComponentMapIdDataObj componentMapId)
throws CovRemoteServiceException_Exception
;
/**
* @param groupSpec
* @param groupId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateGroup", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateGroup")
@ResponseWrapper(localName = "updateGroupResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateGroupResponse")
public void updateGroup(
@WebParam(name = "groupId", targetNamespace = "")
GroupIdDataObj groupId,
@WebParam(name = "groupSpec", targetNamespace = "")
GroupSpecDataObj groupSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param groupId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteGroup", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteGroup")
@ResponseWrapper(localName = "deleteGroupResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteGroupResponse")
public void deleteGroup(
@WebParam(name = "groupId", targetNamespace = "")
GroupIdDataObj groupId)
throws CovRemoteServiceException_Exception
;
/**
* @param projectSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createProject", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateProject")
@ResponseWrapper(localName = "createProjectResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateProjectResponse")
public void createProject(
@WebParam(name = "projectSpec", targetNamespace = "")
ProjectSpecDataObj projectSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param projectSpec
* @param projectId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateProject", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateProject")
@ResponseWrapper(localName = "updateProjectResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateProjectResponse")
public void updateProject(
@WebParam(name = "projectId", targetNamespace = "")
ProjectIdDataObj projectId,
@WebParam(name = "projectSpec", targetNamespace = "")
ProjectSpecDataObj projectSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param projectId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteProject", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteProject")
@ResponseWrapper(localName = "deleteProjectResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteProjectResponse")
public void deleteProject(
@WebParam(name = "projectId", targetNamespace = "")
ProjectIdDataObj projectId)
throws CovRemoteServiceException_Exception
;
/**
* @param roleSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createRole", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateRole")
@ResponseWrapper(localName = "createRoleResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateRoleResponse")
public void createRole(
@WebParam(name = "roleSpec", targetNamespace = "")
RoleSpecDataObj roleSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param roleSpec
* @param roleId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateRole", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateRole")
@ResponseWrapper(localName = "updateRoleResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateRoleResponse")
public void updateRole(
@WebParam(name = "roleId", targetNamespace = "")
RoleIdDataObj roleId,
@WebParam(name = "roleSpec", targetNamespace = "")
RoleSpecDataObj roleSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param roleId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteRole", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteRole")
@ResponseWrapper(localName = "deleteRoleResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteRoleResponse")
public void deleteRole(
@WebParam(name = "roleId", targetNamespace = "")
RoleIdDataObj roleId)
throws CovRemoteServiceException_Exception
;
/**
* @param streamSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createStream", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateStream")
@ResponseWrapper(localName = "createStreamResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateStreamResponse")
public void createStream(
@WebParam(name = "streamSpec", targetNamespace = "")
StreamSpecDataObj streamSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param streamId
* @param streamSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateStream", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateStream")
@ResponseWrapper(localName = "updateStreamResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateStreamResponse")
public void updateStream(
@WebParam(name = "streamId", targetNamespace = "")
StreamIdDataObj streamId,
@WebParam(name = "streamSpec", targetNamespace = "")
StreamSpecDataObj streamSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param streamId
* @param onlyIfEmpty
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteStream", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteStream")
@ResponseWrapper(localName = "deleteStreamResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteStreamResponse")
public void deleteStream(
@WebParam(name = "streamId", targetNamespace = "")
StreamIdDataObj streamId,
@WebParam(name = "onlyIfEmpty", targetNamespace = "")
boolean onlyIfEmpty)
throws CovRemoteServiceException_Exception
;
/**
* @param triageStoreSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createTriageStore", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateTriageStore")
@ResponseWrapper(localName = "createTriageStoreResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateTriageStoreResponse")
public void createTriageStore(
@WebParam(name = "triageStoreSpec", targetNamespace = "")
TriageStoreSpecDataObj triageStoreSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param triageStoreSpec
* @param triageStoreId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateTriageStore", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateTriageStore")
@ResponseWrapper(localName = "updateTriageStoreResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateTriageStoreResponse")
public void updateTriageStore(
@WebParam(name = "triageStoreId", targetNamespace = "")
TriageStoreIdDataObj triageStoreId,
@WebParam(name = "triageStoreSpec", targetNamespace = "")
TriageStoreSpecDataObj triageStoreSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param triageStoreId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteTriageStore", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteTriageStore")
@ResponseWrapper(localName = "deleteTriageStoreResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteTriageStoreResponse")
public void deleteTriageStore(
@WebParam(name = "triageStoreId", targetNamespace = "")
TriageStoreIdDataObj triageStoreId)
throws CovRemoteServiceException_Exception
;
/**
* @param userSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createUser", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateUser")
@ResponseWrapper(localName = "createUserResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateUserResponse")
public void createUser(
@WebParam(name = "userSpec", targetNamespace = "")
UserSpecDataObj userSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param username
* @param userSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateUser", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateUser")
@ResponseWrapper(localName = "updateUserResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateUserResponse")
public void updateUser(
@WebParam(name = "username", targetNamespace = "")
String username,
@WebParam(name = "userSpec", targetNamespace = "")
UserSpecDataObj userSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param username
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteUser", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteUser")
@ResponseWrapper(localName = "deleteUserResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteUserResponse")
public void deleteUser(
@WebParam(name = "username", targetNamespace = "")
String username)
throws CovRemoteServiceException_Exception
;
/**
* @param username
* @return returns com.coverity.ws.v9.UserDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getUser", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetUser")
@ResponseWrapper(localName = "getUserResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetUserResponse")
public UserDataObj getUser(
@WebParam(name = "username", targetNamespace = "")
String username)
throws CovRemoteServiceException_Exception
;
/**
* @param filterSpec
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getStreams", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetStreams")
@ResponseWrapper(localName = "getStreamsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetStreamsResponse")
public List getStreams(
@WebParam(name = "filterSpec", targetNamespace = "")
StreamFilterSpecDataObj filterSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param srcTriageStoreIds
* @param triageStoreId
* @param deleteSourceStores
* @param assignStreamsToTargetStore
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "mergeTriageStores", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.MergeTriageStores")
@ResponseWrapper(localName = "mergeTriageStoresResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.MergeTriageStoresResponse")
public void mergeTriageStores(
@WebParam(name = "srcTriageStoreIds", targetNamespace = "")
List srcTriageStoreIds,
@WebParam(name = "triageStoreId", targetNamespace = "")
TriageStoreIdDataObj triageStoreId,
@WebParam(name = "deleteSourceStores", targetNamespace = "")
boolean deleteSourceStores,
@WebParam(name = "assignStreamsToTargetStore", targetNamespace = "")
boolean assignStreamsToTargetStore)
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.util.List
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getAllPermissions", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAllPermissions")
@ResponseWrapper(localName = "getAllPermissionsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAllPermissionsResponse")
public List getAllPermissions();
/**
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getAllLdapConfigurations", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAllLdapConfigurations")
@ResponseWrapper(localName = "getAllLdapConfigurationsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAllLdapConfigurationsResponse")
public List getAllLdapConfigurations()
throws CovRemoteServiceException_Exception
;
/**
* @param snapshotId
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getDeleteSnapshotJobInfo", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetDeleteSnapshotJobInfo")
@ResponseWrapper(localName = "getDeleteSnapshotJobInfoResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetDeleteSnapshotJobInfoResponse")
public List getDeleteSnapshotJobInfo(
@WebParam(name = "snapshotId", targetNamespace = "")
List snapshotId)
throws CovRemoteServiceException_Exception
;
/**
* @param streamId
* @param filterSpec
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getSnapshotsForStream", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSnapshotsForStream")
@ResponseWrapper(localName = "getSnapshotsForStreamResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSnapshotsForStreamResponse")
public List getSnapshotsForStream(
@WebParam(name = "streamId", targetNamespace = "")
StreamIdDataObj streamId,
@WebParam(name = "filterSpec", targetNamespace = "")
SnapshotFilterSpecDataObj filterSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param serverDomainIdDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteLdapConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteLdapConfiguration")
@ResponseWrapper(localName = "deleteLdapConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteLdapConfigurationResponse")
public void deleteLdapConfiguration(
@WebParam(name = "serverDomainIdDataObj", targetNamespace = "")
ServerDomainIdDataObj serverDomainIdDataObj)
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.util.List
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getLastUpdateTimes", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLastUpdateTimes")
@ResponseWrapper(localName = "getLastUpdateTimesResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLastUpdateTimesResponse")
public List getLastUpdateTimes();
/**
* @param acceptNewCommits
*/
@WebMethod
@RequestWrapper(localName = "setAcceptingNewCommits", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetAcceptingNewCommits")
@ResponseWrapper(localName = "setAcceptingNewCommitsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetAcceptingNewCommitsResponse")
public void setAcceptingNewCommits(
@WebParam(name = "acceptNewCommits", targetNamespace = "")
boolean acceptNewCommits);
/**
* @param snapshotId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "deleteSnapshot", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteSnapshot")
@ResponseWrapper(localName = "deleteSnapshotResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.DeleteSnapshotResponse")
public void deleteSnapshot(
@WebParam(name = "snapshotId", targetNamespace = "")
List snapshotId)
throws CovRemoteServiceException_Exception
;
/**
* @param viewname
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "executeNotification", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.ExecuteNotification")
@ResponseWrapper(localName = "executeNotificationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.ExecuteNotificationResponse")
public void executeNotification(
@WebParam(name = "viewname", targetNamespace = "")
String viewname)
throws CovRemoteServiceException_Exception
;
/**
* @param projectId
* @param sourceStreamId
* @return returns com.coverity.ws.v9.StreamDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "copyStream", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CopyStream")
@ResponseWrapper(localName = "copyStreamResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CopyStreamResponse")
public StreamDataObj copyStream(
@WebParam(name = "projectId", targetNamespace = "")
ProjectIdDataObj projectId,
@WebParam(name = "sourceStreamId", targetNamespace = "")
StreamIdDataObj sourceStreamId)
throws CovRemoteServiceException_Exception
;
/**
* @param streamSpec
* @param projectId
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createStreamInProject", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateStreamInProject")
@ResponseWrapper(localName = "createStreamInProjectResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateStreamInProjectResponse")
public void createStreamInProject(
@WebParam(name = "projectId", targetNamespace = "")
ProjectIdDataObj projectId,
@WebParam(name = "streamSpec", targetNamespace = "")
StreamSpecDataObj streamSpec)
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.lang.String
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getMessageOfTheDay", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetMessageOfTheDay")
@ResponseWrapper(localName = "getMessageOfTheDayResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetMessageOfTheDayResponse")
public String getMessageOfTheDay();
/**
* @param message
*/
@WebMethod
@RequestWrapper(localName = "setMessageOfTheDay", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetMessageOfTheDay")
@ResponseWrapper(localName = "setMessageOfTheDayResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetMessageOfTheDayResponse")
public void setMessageOfTheDay(
@WebParam(name = "message", targetNamespace = "")
String message);
/**
* @param filterSpec
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getProjects", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetProjects")
@ResponseWrapper(localName = "getProjectsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetProjectsResponse")
public List getProjects(
@WebParam(name = "filterSpec", targetNamespace = "")
ProjectFilterSpecDataObj filterSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param filterSpec
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getComponentMaps", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetComponentMaps")
@ResponseWrapper(localName = "getComponentMapsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetComponentMapsResponse")
public List getComponentMaps(
@WebParam(name = "filterSpec", targetNamespace = "")
ComponentMapFilterSpecDataObj filterSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param filterSpec
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getTriageStores", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetTriageStores")
@ResponseWrapper(localName = "getTriageStoresResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetTriageStoresResponse")
public List getTriageStores(
@WebParam(name = "filterSpec", targetNamespace = "")
TriageStoreFilterSpecDataObj filterSpec)
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getCategoryNames", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetCategoryNames")
@ResponseWrapper(localName = "getCategoryNamesResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetCategoryNamesResponse")
public List getCategoryNames()
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getDefectStatuses", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetDefectStatuses")
@ResponseWrapper(localName = "getDefectStatusesResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetDefectStatusesResponse")
public List getDefectStatuses()
throws CovRemoteServiceException_Exception
;
/**
* @param snapshotIds
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getSnapshotInformation", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSnapshotInformation")
@ResponseWrapper(localName = "getSnapshotInformationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSnapshotInformationResponse")
public List getSnapshotInformation(
@WebParam(name = "snapshotIds", targetNamespace = "")
List snapshotIds)
throws CovRemoteServiceException_Exception
;
/**
* @param snapshotId
* @param snapshotData
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateSnapshotInfo", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateSnapshotInfo")
@ResponseWrapper(localName = "updateSnapshotInfoResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateSnapshotInfoResponse")
public void updateSnapshotInfo(
@WebParam(name = "snapshotId", targetNamespace = "")
SnapshotIdDataObj snapshotId,
@WebParam(name = "snapshotData", targetNamespace = "")
SnapshotInfoDataObj snapshotData)
throws CovRemoteServiceException_Exception
;
/**
* @return returns com.coverity.ws.v9.CommitStateDataObj
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getCommitState", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetCommitState")
@ResponseWrapper(localName = "getCommitStateResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetCommitStateResponse")
public CommitStateDataObj getCommitState();
/**
* @return returns java.util.List
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getLdapServerDomains", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLdapServerDomains")
@ResponseWrapper(localName = "getLdapServerDomainsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLdapServerDomainsResponse")
public List getLdapServerDomains();
/**
* @return returns javax.xml.datatype.XMLGregorianCalendar
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getServerTime", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetServerTime")
@ResponseWrapper(localName = "getServerTimeResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetServerTimeResponse")
public XMLGregorianCalendar getServerTime()
throws CovRemoteServiceException_Exception
;
/**
* @return returns com.coverity.ws.v9.ConfigurationDataObj
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getSystemConfig", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSystemConfig")
@ResponseWrapper(localName = "getSystemConfigResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSystemConfigResponse")
public ConfigurationDataObj getSystemConfig();
/**
* @return returns com.coverity.ws.v9.LicenseStateDataObj
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getLicenseState", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLicenseState")
@ResponseWrapper(localName = "getLicenseStateResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLicenseStateResponse")
public LicenseStateDataObj getLicenseState();
/**
* @return returns com.coverity.ws.v9.SnapshotPurgeDetailsObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getSnapshotPurgeDetails", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSnapshotPurgeDetails")
@ResponseWrapper(localName = "getSnapshotPurgeDetailsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSnapshotPurgeDetailsResponse")
public SnapshotPurgeDetailsObj getSnapshotPurgeDetails()
throws CovRemoteServiceException_Exception
;
/**
* @param purgeDetailsSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "setSnapshotPurgeDetails", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetSnapshotPurgeDetails")
@ResponseWrapper(localName = "setSnapshotPurgeDetailsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetSnapshotPurgeDetailsResponse")
public void setSnapshotPurgeDetails(
@WebParam(name = "purgeDetailsSpec", targetNamespace = "")
SnapshotPurgeDetailsObj purgeDetailsSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param ldapConfigurationSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createLdapConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateLdapConfiguration")
@ResponseWrapper(localName = "createLdapConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateLdapConfigurationResponse")
public void createLdapConfiguration(
@WebParam(name = "ldapConfigurationSpec", targetNamespace = "")
LdapConfigurationSpecDataObj ldapConfigurationSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param ldapConfigurationSpec
* @param serverDomainIdDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateLdapConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateLdapConfiguration")
@ResponseWrapper(localName = "updateLdapConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateLdapConfigurationResponse")
public void updateLdapConfiguration(
@WebParam(name = "serverDomainIdDataObj", targetNamespace = "")
ServerDomainIdDataObj serverDomainIdDataObj,
@WebParam(name = "ldapConfigurationSpec", targetNamespace = "")
LdapConfigurationSpecDataObj ldapConfigurationSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param filterSpec
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getDeveloperStreamsProjects", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetDeveloperStreamsProjects")
@ResponseWrapper(localName = "getDeveloperStreamsProjectsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetDeveloperStreamsProjectsResponse")
public List getDeveloperStreamsProjects(
@WebParam(name = "filterSpec", targetNamespace = "")
ProjectFilterSpecDataObj filterSpec)
throws CovRemoteServiceException_Exception
;
/**
* @return returns com.coverity.ws.v9.SignInSettingsDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getSignInConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSignInConfiguration")
@ResponseWrapper(localName = "getSignInConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSignInConfigurationResponse")
public SignInSettingsDataObj getSignInConfiguration()
throws CovRemoteServiceException_Exception
;
/**
* @return returns com.coverity.ws.v9.LoggingConfigurationDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getLoggingConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLoggingConfiguration")
@ResponseWrapper(localName = "getLoggingConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLoggingConfigurationResponse")
public LoggingConfigurationDataObj getLoggingConfiguration()
throws CovRemoteServiceException_Exception
;
/**
* @param loggingConfigurationDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "setLoggingConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetLoggingConfiguration")
@ResponseWrapper(localName = "setLoggingConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetLoggingConfigurationResponse")
public void setLoggingConfiguration(
@WebParam(name = "loggingConfigurationDataObj", targetNamespace = "")
LoggingConfigurationDataObj loggingConfigurationDataObj)
throws CovRemoteServiceException_Exception
;
/**
* @param licenseSpecDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "importLicense", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.ImportLicense")
@ResponseWrapper(localName = "importLicenseResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.ImportLicenseResponse")
public void importLicense(
@WebParam(name = "licenseSpecDataObj", targetNamespace = "")
LicenseSpecDataObj licenseSpecDataObj)
throws CovRemoteServiceException_Exception
;
/**
* @return returns com.coverity.ws.v9.BackupConfigurationDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getBackupConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetBackupConfiguration")
@ResponseWrapper(localName = "getBackupConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetBackupConfigurationResponse")
public BackupConfigurationDataObj getBackupConfiguration()
throws CovRemoteServiceException_Exception
;
/**
* @param backupConfigurationDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "setBackupConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetBackupConfiguration")
@ResponseWrapper(localName = "setBackupConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetBackupConfigurationResponse")
public void setBackupConfiguration(
@WebParam(name = "backupConfigurationDataObj", targetNamespace = "")
BackupConfigurationDataObj backupConfigurationDataObj)
throws CovRemoteServiceException_Exception
;
/**
* @return returns com.coverity.ws.v9.SkeletonizationConfigurationDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getSkeletonizationConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSkeletonizationConfiguration")
@ResponseWrapper(localName = "getSkeletonizationConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetSkeletonizationConfigurationResponse")
public SkeletonizationConfigurationDataObj getSkeletonizationConfiguration()
throws CovRemoteServiceException_Exception
;
/**
* @param skeletonizationConfigurationDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "setSkeletonizationConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetSkeletonizationConfiguration")
@ResponseWrapper(localName = "setSkeletonizationConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetSkeletonizationConfigurationResponse")
public void setSkeletonizationConfiguration(
@WebParam(name = "skeletonizationConfigurationDataObj", targetNamespace = "")
SkeletonizationConfigurationDataObj skeletonizationConfigurationDataObj)
throws CovRemoteServiceException_Exception
;
/**
* @return returns com.coverity.ws.v9.LicenseDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getLicenseConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLicenseConfiguration")
@ResponseWrapper(localName = "getLicenseConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetLicenseConfigurationResponse")
public LicenseDataObj getLicenseConfiguration()
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.lang.String
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getArchitectureAnalysisConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetArchitectureAnalysisConfiguration")
@ResponseWrapper(localName = "getArchitectureAnalysisConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetArchitectureAnalysisConfigurationResponse")
public String getArchitectureAnalysisConfiguration()
throws CovRemoteServiceException_Exception
;
/**
* @param architectureAnalysisConfiguration
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "setArchitectureAnalysisConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetArchitectureAnalysisConfiguration")
@ResponseWrapper(localName = "setArchitectureAnalysisConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.SetArchitectureAnalysisConfigurationResponse")
public void setArchitectureAnalysisConfiguration(
@WebParam(name = "architectureAnalysisConfiguration", targetNamespace = "")
String architectureAnalysisConfiguration)
throws CovRemoteServiceException_Exception
;
/**
* @param signInSettingsDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "updateSignInConfiguration", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateSignInConfiguration")
@ResponseWrapper(localName = "updateSignInConfigurationResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.UpdateSignInConfigurationResponse")
public void updateSignInConfiguration(
@WebParam(name = "signInSettingsDataObj", targetNamespace = "")
SignInSettingsDataObj signInSettingsDataObj)
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getCheckerNames", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetCheckerNames")
@ResponseWrapper(localName = "getCheckerNamesResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetCheckerNamesResponse")
public List getCheckerNames()
throws CovRemoteServiceException_Exception
;
/**
* @return returns com.coverity.ws.v9.VersionDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getVersion", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetVersion")
@ResponseWrapper(localName = "getVersionResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetVersionResponse")
public VersionDataObj getVersion()
throws CovRemoteServiceException_Exception
;
/**
* @param groupSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createGroup", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateGroup")
@ResponseWrapper(localName = "createGroupResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateGroupResponse")
public void createGroup(
@WebParam(name = "groupSpec", targetNamespace = "")
GroupSpecDataObj groupSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param attributeDefinitionSpec
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@RequestWrapper(localName = "createAttribute", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateAttribute")
@ResponseWrapper(localName = "createAttributeResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.CreateAttributeResponse")
public void createAttribute(
@WebParam(name = "attributeDefinitionSpec", targetNamespace = "")
AttributeDefinitionSpecDataObj attributeDefinitionSpec)
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getTypeNames", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetTypeNames")
@ResponseWrapper(localName = "getTypeNamesResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetTypeNamesResponse")
public List getTypeNames()
throws CovRemoteServiceException_Exception
;
/**
* @param attributeDefinitionId
* @return returns com.coverity.ws.v9.AttributeDefinitionDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getAttribute", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAttribute")
@ResponseWrapper(localName = "getAttributeResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAttributeResponse")
public AttributeDefinitionDataObj getAttribute(
@WebParam(name = "attributeDefinitionId", targetNamespace = "")
AttributeDefinitionIdDataObj attributeDefinitionId)
throws CovRemoteServiceException_Exception
;
/**
* @param componentId
* @return returns com.coverity.ws.v9.ComponentDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getComponent", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetComponent")
@ResponseWrapper(localName = "getComponentResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetComponentResponse")
public ComponentDataObj getComponent(
@WebParam(name = "componentId", targetNamespace = "")
ComponentIdDataObj componentId)
throws CovRemoteServiceException_Exception
;
/**
* @param filterSpec
* @param pageSpec
* @return returns com.coverity.ws.v9.GroupsPageDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getGroups", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetGroups")
@ResponseWrapper(localName = "getGroupsResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetGroupsResponse")
public GroupsPageDataObj getGroups(
@WebParam(name = "filterSpec", targetNamespace = "")
GroupFilterSpecDataObj filterSpec,
@WebParam(name = "pageSpec", targetNamespace = "")
PageSpecDataObj pageSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param filterSpec
* @param pageSpec
* @return returns com.coverity.ws.v9.UsersPageDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getUsers", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetUsers")
@ResponseWrapper(localName = "getUsersResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetUsersResponse")
public UsersPageDataObj getUsers(
@WebParam(name = "filterSpec", targetNamespace = "")
UserFilterSpecDataObj filterSpec,
@WebParam(name = "pageSpec", targetNamespace = "")
PageSpecDataObj pageSpec)
throws CovRemoteServiceException_Exception
;
/**
* @param roleId
* @return returns com.coverity.ws.v9.RoleDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getRole", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetRole")
@ResponseWrapper(localName = "getRoleResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetRoleResponse")
public RoleDataObj getRole(
@WebParam(name = "roleId", targetNamespace = "")
RoleIdDataObj roleId)
throws CovRemoteServiceException_Exception
;
/**
* @param groupId
* @return returns com.coverity.ws.v9.GroupDataObj
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getGroup", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetGroup")
@ResponseWrapper(localName = "getGroupResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetGroupResponse")
public GroupDataObj getGroup(
@WebParam(name = "groupId", targetNamespace = "")
GroupIdDataObj groupId)
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.util.List
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getAllRoles", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAllRoles")
@ResponseWrapper(localName = "getAllRolesResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAllRolesResponse")
public List getAllRoles();
/**
* @param message
* @param usernames
* @param subject
* @return returns java.util.List
* @throws CovRemoteServiceException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "notify", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.Notify")
@ResponseWrapper(localName = "notifyResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.NotifyResponse")
public List notify(
@WebParam(name = "usernames", targetNamespace = "")
List usernames,
@WebParam(name = "subject", targetNamespace = "")
String subject,
@WebParam(name = "message", targetNamespace = "")
String message)
throws CovRemoteServiceException_Exception
;
/**
* @return returns java.util.List
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getAttributes", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAttributes")
@ResponseWrapper(localName = "getAttributesResponse", targetNamespace = "http://ws.coverity.com/v9", className = "com.coverity.ws.v9.GetAttributesResponse")
public List getAttributes();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy