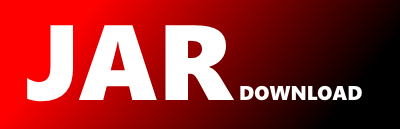
com.synopsys.integration.jira.common.model.response.AvailableAppResponseModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of int-jira-common Show documentation
Show all versions of int-jira-common Show documentation
A library for using various capabilities of Jira.
/**
* int-jira-common
*
* Copyright (c) 2019 Synopsys, Inc.
*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.synopsys.integration.jira.common.model.response;
import java.util.List;
import java.util.Optional;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.synopsys.integration.jira.common.model.JiraResponse;
public class AvailableAppResponseModel extends JiraResponse {
private JsonObject links;
private String key;
private String name;
private JsonObject vendor;
private JsonObject logo;
private String version;
private String license;
private String summary;
private String description;
private JsonObject versionDetails;
private String marketPlaceType;
private Boolean usesLicensing;
private JsonArray categories;
private Double rating;
private Long ratingCount;
private Long reviewCount;
private Long downloadCount;
private Long installationCount;
private Boolean installed;
private Boolean installable;
private Boolean preinstalled;
private Boolean stable;
private Boolean dataCenterCompatible;
private Boolean statusDataCenterCompatible;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy