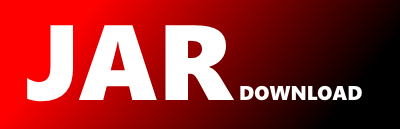
com.synopsys.integration.jira.common.server.configuration.JiraServerRestConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of int-jira-common Show documentation
Show all versions of int-jira-common Show documentation
A library for using various capabilities of Jira.
/*
* int-jira-common
*
* Copyright (c) 2024 Synopsys, Inc.
*
* Use subject to the terms and conditions of the Synopsys End User Software License and Maintenance Agreement. All rights reserved worldwide.
*/
package com.synopsys.integration.jira.common.server.configuration;
import java.net.URL;
import java.util.Optional;
import java.util.function.BiConsumer;
import com.google.gson.Gson;
import com.synopsys.integration.builder.Buildable;
import com.synopsys.integration.jira.common.rest.JiraHttpClient;
import com.synopsys.integration.jira.common.server.service.JiraServerServiceFactory;
import com.synopsys.integration.log.IntLogger;
import com.synopsys.integration.rest.proxy.ProxyInfo;
import com.synopsys.integration.rest.support.AuthenticationSupport;
import com.synopsys.integration.util.Stringable;
import javax.net.ssl.SSLContext;
public abstract class JiraServerRestConfig extends Stringable implements Buildable {
private final URL jiraUrl;
private final int timeoutSeconds;
private final ProxyInfo proxyInfo;
private final boolean alwaysTrustServerCertificate;
private final SSLContext sslContext;
private final Gson gson;
private final AuthenticationSupport authenticationSupport;
protected JiraServerRestConfig(
URL jiraUrl,
int timeoutSeconds,
ProxyInfo proxyInfo,
boolean alwaysTrustServerCertificate,
Gson gson,
AuthenticationSupport authenticationSupport
) {
this.jiraUrl = jiraUrl;
this.timeoutSeconds = timeoutSeconds;
this.proxyInfo = proxyInfo;
this.alwaysTrustServerCertificate = alwaysTrustServerCertificate;
this.sslContext = null;
this.gson = gson;
this.authenticationSupport = authenticationSupport;
}
protected JiraServerRestConfig(
URL jiraUrl,
int timeoutSeconds,
ProxyInfo proxyInfo,
SSLContext sslContext,
Gson gson,
AuthenticationSupport authenticationSupport
) {
this.jiraUrl = jiraUrl;
this.timeoutSeconds = timeoutSeconds;
this.proxyInfo = proxyInfo;
this.alwaysTrustServerCertificate = false;
this.sslContext = sslContext;
this.gson = gson;
this.authenticationSupport = authenticationSupport;
}
public abstract JiraHttpClient createJiraHttpClient(IntLogger logger);
public JiraServerServiceFactory createJiraServerServiceFactory(IntLogger logger) {
return new JiraServerServiceFactory(logger, createJiraHttpClient(logger), gson);
}
public abstract void populateEnvironmentVariables(BiConsumer pairsConsumer);
public URL getJiraUrl() {
return jiraUrl;
}
public int getTimeoutSeconds() {
return timeoutSeconds;
}
public ProxyInfo getProxyInfo() {
return proxyInfo;
}
public boolean isAlwaysTrustServerCertificate() {
return alwaysTrustServerCertificate;
}
public Optional getSslContext() {
return Optional.ofNullable(sslContext);
}
public Gson getGson() {
return gson;
}
public AuthenticationSupport getAuthenticationSupport() {
return authenticationSupport;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy