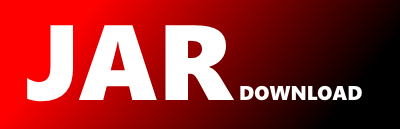
com.sysunite.coinsweb.steps.DocumentReferenceValidation Maven / Gradle / Ivy
The newest version!
package com.sysunite.coinsweb.steps;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.databind.SerializerProvider;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.ser.std.StdSerializer;
import com.sysunite.coinsweb.connector.ConnectorException;
import com.sysunite.coinsweb.filemanager.ContainerFile;
import com.sysunite.coinsweb.graphset.ContainerGraphSet;
import com.sysunite.coinsweb.parser.config.pojo.ConfigPart;
import com.sysunite.coinsweb.parser.config.pojo.GraphVarImpl;
import org.eclipse.rdf4j.query.BindingSet;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.nio.file.Paths;
import java.util.HashMap;
import java.util.List;
import static com.sysunite.coinsweb.parser.Parser.isNotNull;
/**
* @author bastbijl, Sysunite 2017
*/
@JsonInclude(Include.NON_NULL)
public class DocumentReferenceValidation extends ConfigPart implements ValidationStep {
private static final Logger log = LoggerFactory.getLogger(DocumentReferenceValidation.class);
public static final String REFERENCE = "DocumentReferenceValidation";
// Configuration items
private String type = REFERENCE;
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
private GraphVarImpl lookIn;
public GraphVarImpl getLookIn() {
return lookIn;
}
public void setLookIn(GraphVarImpl lookIn) {
this.lookIn = lookIn;
}
// Result items
private boolean failed = true;
public boolean getFailed() {
return failed;
}
public void setFailed(boolean failed) {
this.failed = failed;
}
private boolean valid = false;
public boolean getValid() {
return valid;
}
public void setValid(boolean valid) {
this.valid = valid;
}
@JsonSerialize(using = DocumentReferenceSerializer.class)
private HashMap internalDocumentReferences = new HashMap<>();
public HashMap getInternalDocumentReferences() {
return internalDocumentReferences;
}
public void setInternalDocumentReferences(HashMap internalDocumentReferences) {
this.internalDocumentReferences = internalDocumentReferences;
}
@JsonSerialize(using = DocumentReferenceSerializer.class)
private HashMap unmatchedInternalDocumentReferences = new HashMap<>();
public HashMap getUnmatchedInternalDocumentReferences() {
return unmatchedInternalDocumentReferences;
}
public void setUnmatchedInternalDocumentReferences(HashMap unmatchedInternalDocumentReferences) {
this.unmatchedInternalDocumentReferences = unmatchedInternalDocumentReferences;
}
public void checkConfig() {
isNotNull(lookIn);
}
@Override
public void execute(ContainerFile container, ContainerGraphSet graphSet) {
try {
boolean allReferencesAreSatisfied = true;
if (graphSet.hasContext(getLookIn())) {
String context = graphSet.contextMap().get(getLookIn());
String query =
"PREFIX rdf: " +
"PREFIX cbim: " +
"SELECT ?document ?filePath ?value " +
"FROM NAMED <" + context + "> " +
"WHERE { graph ?g { " +
" ?document rdf:type cbim:InternalDocumentReference . " +
" ?document cbim:filePath ?filePath . " +
" ?filePath cbim:datatypeValue ?value . " +
"}}";
int logCount = 0;
final int MAX_REPORT_COUNT = 50;
final int MAX_LOG_COUNT = 10;
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy