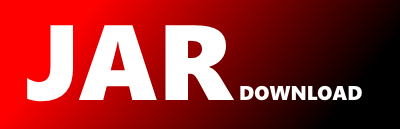
com.sysunite.coinsweb.steps.profile.ValidationExecutor Maven / Gradle / Ivy
The newest version!
/**
* MIT License
*
* Copyright (c) 2016 Bouw Informatie Raad
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the "Software"),
* to deal in the Software without restriction, including without limitation
* the rights to use, copy, modify, merge, publish, distribute, sublicense,
* and/or sell copies of the Software, and to permit persons to whom the
* Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included
* in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
* THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
* IN THE SOFTWARE.
*
**/
package com.sysunite.coinsweb.steps.profile;
import com.sysunite.coinsweb.connector.ConnectorException;
import com.sysunite.coinsweb.graphset.ContainerGraphSet;
import com.sysunite.coinsweb.graphset.GraphVar;
import com.sysunite.coinsweb.graphset.QueryFactory;
import com.sysunite.coinsweb.parser.profile.factory.ProfileFactory;
import com.sysunite.coinsweb.parser.profile.pojo.Bundle;
import com.sysunite.coinsweb.parser.profile.pojo.ProfileFile;
import com.sysunite.coinsweb.parser.profile.pojo.Query;
import com.sysunite.coinsweb.report.ReportFactory;
import com.sysunite.coinsweb.steps.ProfileValidation;
import freemarker.template.Template;
import org.eclipse.rdf4j.model.Value;
import org.eclipse.rdf4j.query.BindingSet;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
/**
* @author Bastiaan Bijl
*/
public class ValidationExecutor {
private static final Logger log = LoggerFactory.getLogger(ValidationExecutor.class);
private ProfileFile profile;
private ContainerGraphSet graphSet;
private ProfileValidation validationConfig;
private String defaultPrefixes = null;
private Map validationGraphs = new HashMap<>();
// // Map context to list of inferenceCode
// Map> executedInferences = new HashMap();
public ValidationExecutor(ProfileFile profile, ContainerGraphSet graphSet, ProfileValidation validationConfig) {
this.profile = profile;
this.graphSet = graphSet;
this.validationConfig = validationConfig;
if(profile.getQueryConfiguration() != null) {
defaultPrefixes = profile.getQueryConfiguration().getDefaultPrefixes();
}
log.info("Using contextMap ("+graphSet.contextMap().keySet().size()+"):");
for(GraphVar graphVar : graphSet.contextMap().keySet()) {
String context = graphSet.contextMap().get(graphVar);
log.info("- " + graphVar + " > "+context);
validationGraphs.put(graphVar.toString(), '<'+context+'>');
}
}
public void validate() {
log.info("Execute profile");
boolean valid = true;
try {
// // Load executed interferences
// for(GraphVar graphVar : graphSet.contextMap().keySet()) {
// String context = graphSet.contextMap().get(graphVar);
// List list = getFinishedInferences(context);
// executedInferences.put(context, list);
// }
// Execute bundles in order of appearance
for (Bundle bundle : profile.getBundles()) {
long start = new Date().getTime();
if (Bundle.INFERENCE.equals(bundle.getType())) {
InferenceBundleStatistics enhanced = executeInferenceBundle(bundle);
validationConfig.addBundle(enhanced);
long executionTime = (new Date().getTime()) - start;
enhanced.addExecutionTimeMs(executionTime);
} else if (Bundle.VALIDATION.equals(bundle.getType())) {
ValidationBundleStatistics enhanced = executeValidationBundle(bundle);
validationConfig.addBundle(enhanced);
valid &= enhanced.getValid();
long executionTime = (new Date().getTime()) - start;
enhanced.addExecutionTimeMs(executionTime);
} else {
throw new RuntimeException("Bundle type " + bundle.getType() + " not supported");
}
}
} catch (ConnectorException e) {
log.error("Executing bundle failed", e);
validationConfig.setFailed(true);
return;
}
validationConfig.setFailed(false);
validationConfig.setValid(valid);
}
private InferenceBundleStatistics executeInferenceBundle(Bundle bundle) throws ConnectorException {
InferenceBundleStatistics bundleStats = new InferenceBundleStatistics(bundle);
String inferenceCode = ProfileFactory.inferenceCode(profile, bundle);
long quadsAddedThisRunSum = 0l;
Map previous = graphSet.quadCount();
Map beforeBundle = previous;
log.info("\uD83D\uDC1A Will perform bundle \""+bundle.getReference()+"\"");
int run = 1;
do {
if(run > validationConfig.getMaxInferenceRuns()) {
throw new RuntimeException("Break running, max number of repeated runs reached for bundle: "+bundle.getReference());
}
long start = new Date().getTime();
for (int i = 0; i < bundleStats.getQueries().size(); i++) {
long startQuery = new Date().getTime();
Query query = bundleStats.getQueries().get(i);
QueryStatistics queryStats = bundleStats.getQuery(query.getReference());
String queryString = QueryFactory.buildQuery(query, validationGraphs, defaultPrefixes);
queryStats.setExecutedQuery(queryString);
try {
graphSet.update(queryString);
} catch (RuntimeException e) {
throw new RuntimeException("Error during execution of update query "+query.getReference() +" of bundle "+bundle.getReference());
}
long executionTimeQuery = (new Date().getTime()) - startQuery;
queryStats.addExecutionTimeMs(executionTimeQuery);
// Do extra run outside executionTime
if(validationConfig.getReportInferenceResults()) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy