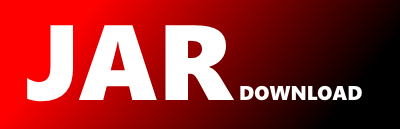
com.tailoredshapes.underbar.Die Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ocho Show documentation
Show all versions of ocho Show documentation
Foundational functions inspired by Clojure and underscore.js
package com.tailoredshapes.underbar;
import com.tailoredshapes.underbar.exceptions.UnderBarred;
import java.util.Collection;
import java.util.Map;
import java.util.function.Supplier;
import java.util.jar.Pack200;
import static com.tailoredshapes.underbar.UnderBar.list;
import static com.tailoredshapes.underbar.function.ExceptionalFunctions.*;
public class Die {
public static T die(Throwable e, String msg, Object... args) {
throw new UnderBarred(args.length == 0 ? msg : String.format(msg, args), e);
}
public static T die(String msg, Object... args) {
throw new UnderBarred(args.length == 0 ? msg : String.format(msg, args));
}
public static void dieIf(boolean condition, Supplier message) {
if (condition) {
die(message.get());
}
}
public static void dieUnless(boolean condition, Supplier message) {
dieIf(!condition, message);
}
public static T dieIfNull(T t, Supplier message) {
if (t == null) {
die(message.get());
}
return t;
}
public static Collection dieIfEmpty(Collection ts, Supplier message) {
dieIf(ts.isEmpty(), message);
return ts;
}
public static Collection dieIfEmpty(T[] tarray, Supplier message) {
return dieIfEmpty(list(tarray), message);
}
public static T dieIfNull(T t) {
return dieIfNull(t, () -> "unexpected null");
}
public static void dieIfNotNull(Object o, Supplier message) {
dieUnless(o == null, message);
}
public static V dieIfMissing(Map map, K key, Supplier message) {
V result = map.get(key);
if (result != null)
return result;
if (map.containsKey(key)) {
die("Key present, but value is null. " + message.get());
}
return die("Key missing: " + message.get());
}
public static V dieIfMissing(Map map, K key) {
return dieIfMissing(map, key, () -> "");
}
public static void rethrow(RunnableWithOops runnable, Supplier errorMessage) {
try {
runnable.run();
} catch (Throwable e) {
die(e, errorMessage.get());
}
}
public static void rethrow(RunnableThatMight runnable, Supplier errorMessage) {
try {
runnable.run();
} catch (Throwable e) {
die(e, errorMessage.get());
}
}
public static void rethrow(RunnableWithOops runnable) {
try {
runnable.run();
} catch (Throwable e) {
die(e, "exception caught");
}
}
public static void rethrow(RunnableThatMight runnable) {
try {
runnable.run();
} catch (Throwable e) {
die(e, "exception caught");
}
}
public static T rethrow(SupplierWithOops supplier) {
try {
return supplier.get();
} catch (Throwable e) {
return die(e, "exception caught");
}
}
public static T rethrow(SupplierThatMight supplier) {
try {
return supplier.get();
} catch (Throwable e) {
return die(e, "exception caught");
}
}
public static T rethrow(SupplierWithOops supplier, Supplier errorMessage) {
try {
return supplier.get();
} catch (Throwable e) {
return die(e, errorMessage.get());
}
}
public static T rethrow(SupplierThatMight supplier, Supplier errorMessage) {
try {
return supplier.get();
} catch (Throwable e) {
return die(e, errorMessage.get());
}
}
public static T wip() {
return die("wip");
}
public static T wip(String reason) {
return die("wip: " + reason);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy