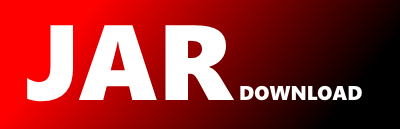
com.talk2object.common.BeanUtils Maven / Gradle / Ivy
package com.talk2object.common;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
/**
* another Bean utils
*
* @author hz00260
*
*/
public class BeanUtils {
/**
* get all fields, including it's super class, until the root class(Object,
* not included).
*
* @param clazz
* @return
*/
public static List getFields(Class clazz) {
List beanFields = new ArrayList();
Class nextClass = clazz;
while (nextClass != Object.class) {
List beanFields2 = getDeclaredFields(nextClass);
beanFields.addAll(beanFields2);
nextClass = nextClass.getSuperclass();
}
return beanFields;
}
/**
* only the declared field, any super class field not included.
* @param clazz
* @return
*/
public static List getDeclaredFields(Class clazz) {
List beanFields = new ArrayList();
Field[] fields = clazz.getDeclaredFields();
for (Field f : fields) {
BeanField bf = new BeanField();
bf.name = f.getName();
Method m;
String s;
try {
s = "get" + makeFirstCharCapital(f.getName());
m = clazz.getMethod(s, null);
bf.getter = s;
} catch (SecurityException e) {
// it is not a public method
// e.printStackTrace();
} catch (NoSuchMethodException e) {
try {
s = "is" + makeFirstCharCapital(f.getName());
m = clazz.getMethod(s, null);
bf.getter = s;
} catch (SecurityException e1) {
// e1.printStackTrace();
} catch (NoSuchMethodException e1) {
// e1.printStackTrace();
}
}
try {
s = "set" + makeFirstCharCapital(f.getName());
m = clazz.getMethod(s, f.getType());
bf.setter = s;
} catch (SecurityException e1) {
// e1.printStackTrace();
} catch (NoSuchMethodException e1) {
// e1.printStackTrace();
}
beanFields.add(bf);
}
return beanFields;
}
private static String makeFirstCharCapital(String s) {
return s.substring(0, 1).toUpperCase() + s.substring(1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy