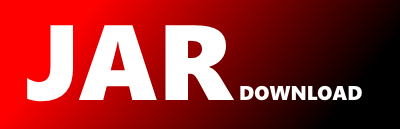
com.talk2object.plum.interaction.rich.field.InteractiveField Maven / Gradle / Ivy
package com.talk2object.plum.interaction.rich.field;
import java.util.ArrayList;
import java.util.List;
import com.talk2object.plum.interaction.input.validation.ValidationPolicy;
import com.talk2object.plum.interaction.input.validation.Validator;
import com.talk2object.plum.interaction.viewgeneneration.ViewPrivillege;
/**
* It is abstract interactive object, which describes prompt text, validation
* rule etc. Later, a concrete interaction manager can use these information to
* render a GUI object.
*
* other informations: options(for enumeration), required, hint, etc.
*
* @author jackding
*
*/
public class InteractiveField {
protected String name;
/**
* the class of field or parameter.
*/
protected Class fieldClass;
protected FieldType type;
protected ViewPrivillege privillege;
protected Object initValue;
/**
* optional
*/
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy