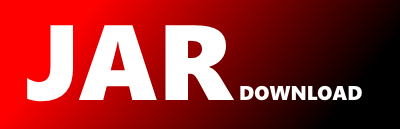
com.talk2object.plum.view._abstract.components.Pagingnation Maven / Gradle / Ivy
package com.talk2object.plum.view._abstract.components;
public class Pagingnation {
private int pageSize;
private long totalSize;
/**
* the max page numbers listed on UI
*/
private int maxListedPageCount = 10;
private int currentPage = 1;
public Pagingnation(int pageSize, long totalSize) {
this.pageSize = pageSize;
this.totalSize = totalSize;
}
public void setCurrentPage(int currentPage) {
this.currentPage = currentPage;
}
public int[] getListedPages() {
// by default, the current page number is at the center position
int min = currentPage - maxListedPageCount / 2;
int max = min + maxListedPageCount - 1;
if (min < 1) {
// shift to right
min = getFirstPage();
max = maxListedPageCount;
if (max > getLastPage())
max = getLastPage();
}
if (max > getLastPage()) {
// shift to left
max = getLastPage();
min = max - maxListedPageCount;
if (min < getFirstPage())
min = getFirstPage();
}
int listedPageSize = (int) (max - min + 1);
int[] pages = new int[listedPageSize];
for (int i = 0; i < listedPageSize; i++) {
pages[i] = min + i;
}
return pages;
}
public int getPreviousPage() {
return currentPage - 1;
}
public int getNextPage() {
return currentPage + 1;
}
public int getFirstPage() {
return 1;
}
public int getLastPage() {
return (int) (((totalSize - 1) / pageSize) + 1);
}
public void gotoFirstPage() {
setCurrentPage(getFirstPage());
}
public void gotoLastPage() {
setCurrentPage(getLastPage());
}
public void gotoPreviousPage() {
setCurrentPage(getPreviousPage());
}
public void gotoNextPage() {
setCurrentPage(getNextPage());
}
public long getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
public int getCurrentPage() {
return currentPage;
}
public boolean isValidPage(int page) {
return page >= 1 && page <= getLastPage();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy