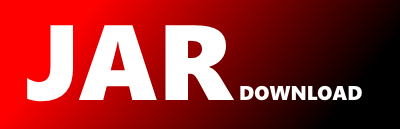
com.talk2object.plum.view._abstract.components.grid.EntityGrid Maven / Gradle / Ivy
package com.talk2object.plum.view._abstract.components.grid;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.beanutils.PropertyUtils;
import com.talk2object.plum.interaction.Form;
import com.talk2object.plum.interaction.InteractionManager;
import com.talk2object.plum.interaction.annotation.parameter.helper.ParameterWrapperFactory;
import com.talk2object.plum.interaction.context.InteractionContextUtils;
import com.talk2object.plum.interaction.rich.field.InteractiveField;
import com.talk2object.plum.interaction.rich.field.ValueType;
import com.talk2object.plum.model.entity.Entity;
import com.talk2object.plum.model.entity.EntityCollection;
import com.talk2object.plum.model.entity.Field;
import com.talk2object.plum.repository.biz.interafce.Collection;
import com.talk2object.plum.repository.biz.interafce.Identifiable;
import com.talk2object.plum.view.Visitor;
import com.talk2object.plum.view._abstract.components.PageChangedListener;
import com.talk2object.plum.view._abstract.components.PagingationPanel;
import com.talk2object.plum.view._abstract.components.Pagingnation;
import com.talk2object.plum.view.component.Button;
import com.talk2object.plum.view.component.CheckBox;
import com.talk2object.plum.view.component.ClickEventListener;
import com.talk2object.plum.view.component.Component;
import com.talk2object.plum.view.component.Label;
import com.talk2object.plum.view.component.containers.layout.Grid;
import com.talk2object.plum.view.component.containers.layout.HorizontalBox;
import com.talk2object.plum.view.component.containers.layout.VerticalBox;
import com.talk2object.plum.view.component.containers.window.ModalResult;
import com.talk2object.plum.view.event.ValueChangeListener;
/**
* it is a view component for one type of entity. the repository provides
* interface of CRUD.
*
* @author jack
*
*/
public class EntityGrid extends VerticalBox {
private Pagingnation pagingation;
private Grid layout;
private HorizontalBox actionPanel;
private PagingationPanel pagingnationPanel;
Map checkBoxMap = new HashMap();
// model
Entity entityMeta;
Collection collection;
public void setEntityCollectionModel(EntityCollection model) {
setModel(model);
}
@Override
public void updateView() {
super.updateView();
EntityCollection model = (EntityCollection) getModel();
entityMeta = model.getMeta();
collection = model.getCollection();
int cols = entityMeta.getFieldSize();
long rows = collection.size();
// extra col is for operations
layout = new Grid(cols + 1);
layout.setHeaderEnabled(true);
addChild(layout);
actionPanel = new HorizontalBox();
addChild(actionPanel);
pagingation = new Pagingnation(10, collection.size());
createActionPanel();
refreshGrid();
}
private void createActionPanel() {
// create action panel
final CheckBox checkAll = new CheckBox();
checkAll.setCaption("Check All");
checkAll.addEventListener(EVENT_VALUE_CHANGE,
new ValueChangeListener() {
@Override
public void onValueChange(Boolean oldValue, Boolean newValue) {
// check all
for (CheckBox cb : checkBoxMap.keySet()) {
cb.setChecked(newValue);
}
}
});
actionPanel.addChild(checkAll);
Button deleteSelectedEntitiesButton = new Button();
deleteSelectedEntitiesButton.setCaption("Delete");
deleteSelectedEntitiesButton.addClickListener(new ClickEventListener() {
@Override
public void onClick(Component source) {
int changedCount = 0;
// delete selected items
for (CheckBox cb : checkBoxMap.keySet()) {
if (cb.isChecked()) {
T entity = checkBoxMap.get(cb);
collection.remove(entity);
changedCount++;
}
}
checkAll.setChecked(false);
if (changedCount > 0)
refreshGrid();
}
});
actionPanel.addChild(deleteSelectedEntitiesButton);
Button createButton = new Button();
createButton.setCaption("Create");
createButton.addClickListener(new ClickEventListener() {
@Override
public void onClick(Component source) {
InteractionManager manager = InteractionContextUtils
.getInteractionManager();
try {
T entity = entityMeta.createNewInstance();
List inputs = createInputs(entity);
Form form = manager.scan(entityMeta.getName(), inputs);
if (ModalResult.OK == form.getModalResult()) {
updateEntity(inputs, entity);
collection.add(entity);
refreshGrid();
}
} catch (Exception e) {
throw new RuntimeException(e);
}
}
});
actionPanel.addChild(createButton);
createPagination();
actionPanel.addChild(pagingnationPanel);
}
/**
* First | Prev | page no here.... | Next | Last
*/
private void createPagination() {
pagingnationPanel = new PagingationPanel(pagingation);
pagingnationPanel
.addPageChangedEventListener(new PageChangedListener() {
@Override
public void onPageChanged(int page) {
refreshGrid();
}
});
}
public void refreshGrid() {
removeChild(layout);
checkBoxMap.clear();
int cols = entityMeta.getFieldSize();
// int rows = collection.size();
Label label;
// extra col is for operations
layout = new Grid(cols + 1);
layout.setHeaderEnabled(true);
addChild(layout);
List entities = collection.getByPage(
(int) pagingation.getPageSize(),
(int) pagingation.getCurrentPage());
// head
label = new Label();
label.setText("Action");
layout.addChild(label);
for (Field field : entityMeta.getFields()) {
label = new Label();
label.setText(field.getName());
layout.addChild(label);
}
for (int r = 0; r < entities.size(); r++) {
final T entity = entities.get(r);
createActionColumn(entity);
for (int c = 0; c < cols; c++) {
Field fieldMeta = entityMeta.getField(c);
Object fieldValue;
try {
fieldValue = PropertyUtils.getProperty(entity,
fieldMeta.getName());
} catch (Exception e) {
throw new RuntimeException(e);
}
label = new Label();
label.setText(obj2String(fieldValue));
layout.addChild(label);
}
}
}
private String obj2String(Object obj) {
if (obj == null)
return "";
else
return obj.toString();
}
private void createActionColumn(final T entity) {
HorizontalBox actionBox = new HorizontalBox();
layout.addChild(actionBox);
CheckBox checkBox = new CheckBox();
checkBox.setCaption("");
actionBox.addChild(checkBox);
// add to map for later query
checkBoxMap.put(checkBox, entity);
// col of operation
Button deleteBtn = new Button();
deleteBtn.setCaption("Delete");
deleteBtn.addClickListener(new ClickEventListener() {
@Override
public void onClick(Component source) {
InteractionManager manager = InteractionContextUtils
.getInteractionManager();
if (manager.confirm("Are you sure to delete it?") == ModalResult.YES) {
collection.remove(entity);
refreshGrid();
}
}
});
actionBox.addChild(deleteBtn);
Button updateBtn = new Button();
updateBtn.setCaption("Update");
updateBtn.addClickListener(new ClickEventListener() {
@Override
public void onClick(Component source) {
InteractionManager manager = InteractionContextUtils
.getInteractionManager();
List inputs = createInputs(entity);
Form form = manager.scan(entityMeta.getName(), inputs);
if (ModalResult.OK == form.getModalResult()) {
updateEntity(inputs, entity);
collection.update(entity);
refreshGrid();
}
}
});
actionBox.addChild(updateBtn);
}
private List createInputs(Identifiable entity) {
List inputs = new ArrayList();
for (Field metaField : entityMeta.getFields()) {
Class fieldClass = metaField.getType();
ValueType type = ValueType.valueOf(fieldClass);
String name = metaField.getName();
InteractiveField input = type.createRichField(name, fieldClass,
null);
ParameterWrapperFactory.getInstance().processAnnotations(input,
metaField.getReflectionField().getAnnotations());
try {
Object value = PropertyUtils.getProperty(entity, name);
input.setInitValue(value);
} catch (Exception e) {
throw new RuntimeException(e);
}
inputs.add(input);
}
return inputs;
}
private void updateEntity(List inputs, Identifiable entity) {
for (InteractiveField input : inputs) {
try {
PropertyUtils.setProperty(entity, input.getName(),
input.getValue());
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
public Pagingnation getPagingation() {
return pagingation;
}
@Override
public Object accept(Visitor visitor) {
return visitor.visit(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy