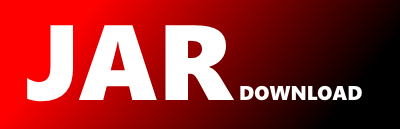
com.taobao.arthas.bytekit.utils.AsmAnnotationUtils Maven / Gradle / Ivy
package com.taobao.arthas.bytekit.utils;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import com.alibaba.arthas.deps.org.objectweb.asm.tree.AnnotationNode;
/**
*
* @author hengyunabc 2020-05-04
*
*/
public class AsmAnnotationUtils {
public static List queryAnnotationInfo(List annotations, String annotationType,
String key) {
List result = new ArrayList();
if (annotations != null) {
for (AnnotationNode annotationNode : annotations) {
if (annotationNode.desc.equals(annotationType)) {
if (annotationNode.values != null) {
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy