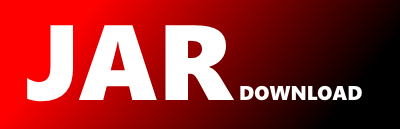
com.alibaba.fastjson.JSONObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastjson Show documentation
Show all versions of fastjson Show documentation
Fastjson is a JSON processor (JSON parser + JSON generator) written in Java
The newest version!
/*
* Copyright 1999-2017 Alibaba Group.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.alibaba.fastjson;
import static com.alibaba.fastjson.util.TypeUtils.castToBigDecimal;
import static com.alibaba.fastjson.util.TypeUtils.castToBigInteger;
import static com.alibaba.fastjson.util.TypeUtils.castToBoolean;
import static com.alibaba.fastjson.util.TypeUtils.castToByte;
import static com.alibaba.fastjson.util.TypeUtils.castToBytes;
import static com.alibaba.fastjson.util.TypeUtils.castToDate;
import static com.alibaba.fastjson.util.TypeUtils.castToDouble;
import static com.alibaba.fastjson.util.TypeUtils.castToFloat;
import static com.alibaba.fastjson.util.TypeUtils.castToInt;
import static com.alibaba.fastjson.util.TypeUtils.castToLong;
import static com.alibaba.fastjson.util.TypeUtils.castToShort;
import static com.alibaba.fastjson.util.TypeUtils.castToSqlDate;
import static com.alibaba.fastjson.util.TypeUtils.castToTimestamp;
import java.io.*;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.*;
import com.alibaba.fastjson.annotation.JSONField;
import com.alibaba.fastjson.parser.Feature;
import com.alibaba.fastjson.parser.ParserConfig;
import com.alibaba.fastjson.util.TypeUtils;
/**
* @author wenshao[[email protected]]
*/
public class JSONObject extends JSON implements Map, Cloneable, Serializable, InvocationHandler {
private static final long serialVersionUID = 1L;
private static final int DEFAULT_INITIAL_CAPACITY = 16;
private final Map map;
public JSONObject(){
this(DEFAULT_INITIAL_CAPACITY, false);
}
public JSONObject(Map map){
if (map == null) {
throw new IllegalArgumentException("map is null.");
}
this.map = map;
}
public JSONObject(boolean ordered){
this(DEFAULT_INITIAL_CAPACITY, ordered);
}
public JSONObject(int initialCapacity){
this(initialCapacity, false);
}
public JSONObject(int initialCapacity, boolean ordered){
if (ordered) {
map = new LinkedHashMap(initialCapacity);
} else {
map = new HashMap(initialCapacity);
}
}
public int size() {
return map.size();
}
public boolean isEmpty() {
return map.isEmpty();
}
public boolean containsKey(Object key) {
boolean result = map.containsKey(key);
if (!result) {
if (key instanceof Number
|| key instanceof Character
|| key instanceof Boolean
|| key instanceof UUID
) {
result = map.containsKey(key.toString());
}
}
return result;
}
public boolean containsValue(Object value) {
return map.containsValue(value);
}
public Object get(Object key) {
Object val = map.get(key);
if (val == null) {
if (key instanceof Number
|| key instanceof Character
|| key instanceof Boolean
|| key instanceof UUID
) {
val = map.get(key.toString());
}
}
return val;
}
public Object getOrDefault(Object key, Object defaultValue) {
Object v;
return ((v = get(key)) != null) ? v : defaultValue;
}
public JSONObject getJSONObject(String key) {
Object value = map.get(key);
if (value instanceof JSONObject) {
return (JSONObject) value;
}
if (value instanceof Map) {
return new JSONObject((Map) value);
}
if (value instanceof String) {
return JSON.parseObject((String) value);
}
return (JSONObject) toJSON(value);
}
public JSONArray getJSONArray(String key) {
Object value = map.get(key);
if (value instanceof JSONArray) {
return (JSONArray) value;
}
if (value instanceof List) {
return new JSONArray((List) value);
}
if (value instanceof String) {
return (JSONArray) JSON.parse((String) value);
}
return (JSONArray) toJSON(value);
}
public T getObject(String key, Class clazz) {
Object obj = map.get(key);
return TypeUtils.castToJavaBean(obj, clazz);
}
public T getObject(String key, Type type) {
Object obj = map.get(key);
return TypeUtils.cast(obj, type, ParserConfig.getGlobalInstance());
}
public T getObject(String key, TypeReference typeReference) {
Object obj = map.get(key);
if (typeReference == null) {
return (T) obj;
}
return TypeUtils.cast(obj, typeReference.getType(), ParserConfig.getGlobalInstance());
}
public Boolean getBoolean(String key) {
Object value = get(key);
if (value == null) {
return null;
}
return castToBoolean(value);
}
public byte[] getBytes(String key) {
Object value = get(key);
if (value == null) {
return null;
}
return castToBytes(value);
}
public boolean getBooleanValue(String key) {
Object value = get(key);
Boolean booleanVal = castToBoolean(value);
if (booleanVal == null) {
return false;
}
return booleanVal.booleanValue();
}
public Byte getByte(String key) {
Object value = get(key);
return castToByte(value);
}
public byte getByteValue(String key) {
Object value = get(key);
Byte byteVal = castToByte(value);
if (byteVal == null) {
return 0;
}
return byteVal.byteValue();
}
public Short getShort(String key) {
Object value = get(key);
return castToShort(value);
}
public short getShortValue(String key) {
Object value = get(key);
Short shortVal = castToShort(value);
if (shortVal == null) {
return 0;
}
return shortVal.shortValue();
}
public Integer getInteger(String key) {
Object value = get(key);
return castToInt(value);
}
public int getIntValue(String key) {
Object value = get(key);
Integer intVal = castToInt(value);
if (intVal == null) {
return 0;
}
return intVal.intValue();
}
public Long getLong(String key) {
Object value = get(key);
return castToLong(value);
}
public long getLongValue(String key) {
Object value = get(key);
Long longVal = castToLong(value);
if (longVal == null) {
return 0L;
}
return longVal.longValue();
}
public Float getFloat(String key) {
Object value = get(key);
return castToFloat(value);
}
public float getFloatValue(String key) {
Object value = get(key);
Float floatValue = castToFloat(value);
if (floatValue == null) {
return 0F;
}
return floatValue.floatValue();
}
public Double getDouble(String key) {
Object value = get(key);
return castToDouble(value);
}
public double getDoubleValue(String key) {
Object value = get(key);
Double doubleValue = castToDouble(value);
if (doubleValue == null) {
return 0D;
}
return doubleValue.doubleValue();
}
public BigDecimal getBigDecimal(String key) {
Object value = get(key);
return castToBigDecimal(value);
}
public BigInteger getBigInteger(String key) {
Object value = get(key);
return castToBigInteger(value);
}
public String getString(String key) {
Object value = get(key);
if (value == null) {
return null;
}
return value.toString();
}
public Date getDate(String key) {
Object value = get(key);
return castToDate(value);
}
public Object getSqlDate(String key) {
Object value = get(key);
return castToSqlDate(value);
}
public Object getTimestamp(String key) {
Object value = get(key);
return castToTimestamp(value);
}
public Object put(String key, Object value) {
return map.put(key, value);
}
public JSONObject fluentPut(String key, Object value) {
map.put(key, value);
return this;
}
public void putAll(Map extends String, ?> m) {
map.putAll(m);
}
public JSONObject fluentPutAll(Map extends String, ?> m) {
map.putAll(m);
return this;
}
public void clear() {
map.clear();
}
public JSONObject fluentClear() {
map.clear();
return this;
}
public Object remove(Object key) {
return map.remove(key);
}
public JSONObject fluentRemove(Object key) {
map.remove(key);
return this;
}
public Set keySet() {
return map.keySet();
}
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy