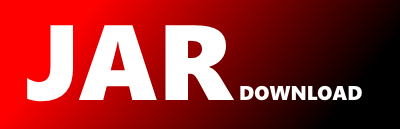
com.alibaba.arthas.nat.agent.proxy.server.handler.RequestHandler Maven / Gradle / Ivy
package com.alibaba.arthas.nat.agent.proxy.server.handler;
import com.alibaba.arthas.nat.agent.proxy.server.handler.http.HttpRequestHandler;
import com.alibaba.arthas.nat.agent.proxy.server.handler.ws.WsRequestHandler;
import io.netty.channel.Channel;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.handler.codec.http.FullHttpRequest;
import io.netty.handler.codec.http.HttpHeaderNames;
import io.netty.handler.codec.http.websocketx.WebSocketFrame;
/**
* @description: RequestHandler
* @author:flzjkl
* @date: 2024-10-19 9:34
*/
public class RequestHandler extends SimpleChannelInboundHandler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy