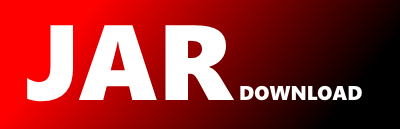
com.taobao.tdhs.client.net.ConnectionPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tdhs-java-client Show documentation
Show all versions of tdhs-java-client Show documentation
A TDH_SOCKET Client For Java
/*
* Copyright(C) 2011-2012 Alibaba Group Holding Limited
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License version 2 as
* published by the Free Software Foundation.
*
* Authors:
* wentong
*/
package com.taobao.tdhs.client.net;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
/**
* @author 文通
* @since 11-12-26 下午3:55
*/
public class ConnectionPool {
private List pool;
private int index = 0;
private ReadWriteLock rwLock = new ReentrantReadWriteLock();
public ConnectionPool(int num) {
pool = new ArrayList(num);
}
public void add(T t, Handler handler) {
rwLock.writeLock().lock();
try {
if (handler != null) {
handler.execute(t);
}
pool.add(t);
} finally {
rwLock.writeLock().unlock();
}
}
public boolean remove(T t) {
rwLock.writeLock().lock();
try {
return pool.remove(t);
} finally {
rwLock.writeLock().unlock();
}
}
/**
* Method get ...
*
* @return Channel
*/
public T get() {
rwLock.readLock().lock();
try {
if (pool.isEmpty()) {
return null;
}
int idx = Math.abs(index++ % pool.size());
return pool.get(idx);
} finally {
rwLock.readLock().unlock();
}
}
public void close(Handler handler) {
rwLock.writeLock().lock();
try {
Iterator iterator = pool.iterator();
while (iterator.hasNext()) {
T t = iterator.next();
if (handler != null) {
handler.execute(t);
}
iterator.remove();
}
} finally {
rwLock.writeLock().unlock();
}
}
public int size() {
rwLock.readLock().lock();
try {
return pool.size();
} finally {
rwLock.readLock().unlock();
}
}
public boolean isEmpty() {
rwLock.readLock().lock();
try {
return pool.isEmpty();
} finally {
rwLock.readLock().unlock();
}
}
public interface Handler {
void execute(T t);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy