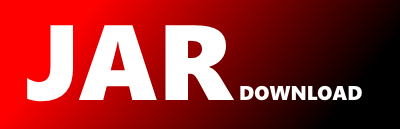
com.taosdata.jdbc.tmq.ConsumerRecords Maven / Gradle / Ivy
package com.taosdata.jdbc.tmq;
import java.util.*;
public class ConsumerRecords implements Iterable {
public static final ConsumerRecords> EMPTY = new ConsumerRecords(Collections.EMPTY_MAP);
private final Map> records;
public ConsumerRecords(Map> records) {
this.records = records;
}
public List records(TopicPartition partition) {
List recs = this.records.get(partition);
if (recs == null)
return Collections.emptyList();
else
return Collections.unmodifiableList(recs);
}
@Override
public Iterator iterator() {
return new Iterator() {
final Iterator extends Iterable> iters = records.values().iterator();
Iterator current;
@Override
public boolean hasNext() {
while (current == null || !current.hasNext()) {
if (iters.hasNext())
current = iters.next().iterator();
else
return false;
}
return true;
}
@Override
public V next() {
return current.next();
}
};
}
public boolean isEmpty() {
return records.isEmpty();
}
@SuppressWarnings("rawtypes")
public static ConsumerRecords empty() {
return EMPTY;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy