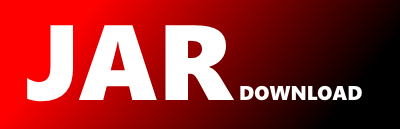
com.taosdata.jdbc.common.ColumnInfo Maven / Gradle / Ivy
package com.taosdata.jdbc.common;
import java.util.ArrayList;
import java.util.List;
public class ColumnInfo implements Comparable {
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy