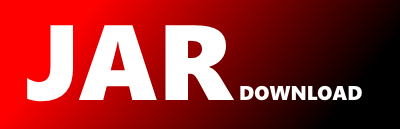
com.taosdata.jdbc.utils.CompletableFutureTimeout Maven / Gradle / Ivy
package com.taosdata.jdbc.utils;
import com.taosdata.jdbc.TSDBError;
import java.util.concurrent.*;
import java.util.function.Function;
import static com.taosdata.jdbc.TSDBErrorNumbers.ERROR_QUERY_TIMEOUT;
public class CompletableFutureTimeout {
private CompletableFutureTimeout() {
}
public static CompletableFuture orTimeout(CompletableFuture future, long timeout, TimeUnit unit, String msg) {
final CompletableFuture timeoutFuture = timeoutAfter(timeout, unit, msg);
return future.applyToEither(timeoutFuture, Function.identity());
}
// return the default value when exception
public static CompletableFuture orTimeout(T t, CompletableFuture future, long timeout, TimeUnit unit, String msg) {
final CompletableFuture timeoutFuture = timeoutAfter(timeout, unit, msg);
return future.applyToEither(timeoutFuture, Function.identity()).exceptionally(throwable -> t);
}
private static CompletableFuture timeoutAfter(long timeout, TimeUnit unit, String msg) {
CompletableFuture result = new CompletableFuture<>();
Delayer.delayer.schedule(
() -> result.completeExceptionally(TSDBError.createTimeoutException(ERROR_QUERY_TIMEOUT,
String.format("failed to complete the task:%s within the specified time : %d,%s", msg, timeout, unit)))
, timeout, unit);
return result;
}
/**
* Singleton delay scheduler, used only for starting and * cancelling tasks.
*/
static final class Delayer {
private Delayer() {
}
@SuppressWarnings("all")
static ScheduledFuture> delay(Runnable command, long delay,
TimeUnit unit) {
return delayer.schedule(command, delay, unit);
}
static final class DaemonThreadFactory implements ThreadFactory {
@Override
public Thread newThread(Runnable r) {
Thread t = new Thread(r);
t.setDaemon(true);
t.setName("DelayScheduler-");
return t;
}
}
static final ScheduledThreadPoolExecutor delayer;
static {
delayer = new ScheduledThreadPoolExecutor(
1, new DaemonThreadFactory());
delayer.setRemoveOnCancelPolicy(true);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy