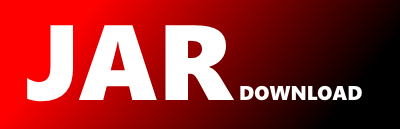
com.taosdata.jdbc.common.Consumer Maven / Gradle / Ivy
package com.taosdata.jdbc.common;
import com.taosdata.jdbc.tmq.*;
import java.sql.SQLException;
import java.time.Duration;
import java.util.Collection;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
public interface Consumer {
/**
* create consumer
*
* @param properties ip / port / user / password and so on.
* @throws SQLException jni exception
*/
void create(Properties properties) throws SQLException;
/**
* subscribe topics
*
* @param topics collection of topics
*/
void subscribe(Collection topics) throws SQLException;
/**
* unsubscribe topics
*/
void unsubscribe() throws SQLException;
/**
* get subscribe topics
*
* @return topic set
*/
Set subscription() throws SQLException;
/**
* get result records
*
* @param timeout wait time for poll data
* @param deserializer convert resultSet to javaBean
* @return ConsumerRecord is the collection of javaBean
* @throws SQLException java reflect exception or resultSet convert exception
*/
ConsumerRecords poll(Duration timeout, Deserializer deserializer) throws SQLException;
/**
* commit offset with sync
*/
void commitSync() throws SQLException;
/**
* close consumer
*/
void close() throws SQLException;
void commitAsync(OffsetCommitCallback callback) throws SQLException;
/**
* If this API is invoked for the same partition more than once, the latest offset will be used on the next poll().
*/
void seek(TopicPartition partition, long offset) throws SQLException;
/**
* Get the offset of the next record that will be fetched.
*/
long position(TopicPartition partition) throws SQLException;
Map position(String topic) throws SQLException;
Map beginningOffsets(String topic) throws SQLException;
Map endOffsets(String topic) throws SQLException;
void seekToBeginning(Collection partitions) throws SQLException;
void seekToEnd(Collection partitions) throws SQLException;
/**
* Get the set of partitions currently assigned to this consumer.
*/
Set assignment() throws SQLException;
/**
* Get the last committed offset for the given partition.
* This offset will be used as the position for the consumer in the event of a failure.
*/
OffsetAndMetadata committed(TopicPartition partition) throws SQLException;
Map committed(final Set partitions) throws SQLException;
void commitSync(final Map offsets) throws SQLException;
void commitAsync(final Map offsets, OffsetCommitCallback callback);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy