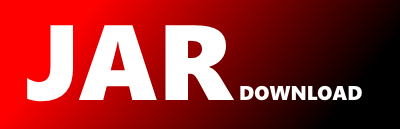
com.tapstream.sdk.TimelineSummaryResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tapstream-core Show documentation
Show all versions of tapstream-core Show documentation
This library contains the building blocks for a Tapstream Java SDK
package com.tapstream.sdk;
import com.tapstream.sdk.http.HttpResponse;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* Created by adam on 2016-10-13.
*/
public class TimelineSummaryResponse implements ApiResponse {
private final HttpResponse httpResponse;
private final String latestDeeplink;
private final List deeplinks;
private final List campaigns;
private final Map hitParams;
private final Map eventParams;
private static List jsonArrayToStringList(JSONArray arr) {
List strs = new ArrayList(arr.length());
if(arr != null) {
for (int ii = 0; ii < arr.length(); ii++) {
strs.add(arr.getString(ii));
}
}
return strs;
}
private static Map jsonObjectToStringMap(JSONObject obj){
Map strs = new HashMap(obj.length());
if(obj != null) {
Iterator ks = obj.keys();
while(ks.hasNext()) {
String k = (String) ks.next();
strs.put(k, obj.getString(k));
}
}
return strs;
}
static TimelineSummaryResponse createSummaryResponse(HttpResponse response) {
String rawResponse = response.getBodyAsString();
try {
if (rawResponse != null) {
JSONObject asJson = new JSONObject(rawResponse);
String latestDeeplink = asJson.getString("latest_deeplink");
List deeplinks = jsonArrayToStringList(asJson.getJSONArray("deeplinks"));
List campaigns = jsonArrayToStringList(asJson.getJSONArray("campaigns"));
Map hitParams = jsonObjectToStringMap(asJson.getJSONObject("hit_params"));
Map eventParams = jsonObjectToStringMap(asJson.getJSONObject("event_params"));
return new TimelineSummaryResponse(response, latestDeeplink, deeplinks, campaigns, hitParams, eventParams);
}
}catch(JSONException e){
Logging.log(Logging.WARN, "JSON decode error from timeline summary: (%s)", e.getMessage());
}
return new TimelineSummaryResponse(response);
}
public TimelineSummaryResponse(HttpResponse httpResponse){
this.httpResponse = httpResponse;
this.latestDeeplink = null;
this.deeplinks = null;
this.campaigns = null;
this.hitParams = null;
this.eventParams = null;
}
public TimelineSummaryResponse(HttpResponse httpResponse, String latestDeeplink, List deeplinks, List campaigns, Map hitParams, Map eventParams) {
this.httpResponse = httpResponse;
this.latestDeeplink = latestDeeplink;
this.deeplinks = deeplinks;
this.campaigns = campaigns;
this.hitParams = hitParams;
this.eventParams = eventParams;
}
@Override
public HttpResponse getHttpResponse() {
return httpResponse;
}
public boolean isEmpty() {
return (eventParams == null || eventParams.isEmpty())
&& (campaigns == null || campaigns.isEmpty()); // Sufficient
}
public String getLatestDeeplink() {
return latestDeeplink;
}
public List getDeeplinks() {
return deeplinks;
}
public List getCampaigns() {
return campaigns;
}
public Map getHitParams() {
return hitParams;
}
public Map getEventParams() {
return eventParams;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy