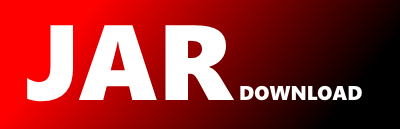
com.targomo.client.api.TravelOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for easy usage of Targomo web services.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy