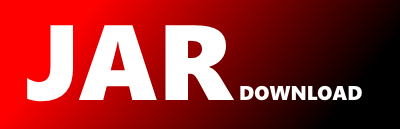
com.targomo.client.api.util.CollectionUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for easy usage of Targomo web services.
The newest version!
package com.targomo.client.api.util;
import com.targomo.client.api.exception.TargomoClientRuntimeException;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.NavigableSet;
import java.util.concurrent.ConcurrentSkipListSet;
public class CollectionUtils {
public static Map map(Object... objects) {
if (objects == null || objects.length % 2 != 0) {
throw new TargomoClientRuntimeException("Key or value is missing");
}
Map map = new LinkedHashMap<>();
for (int i = 0; i < objects.length; i += 2) {
map.put((K) objects[i], (V) objects[i + 1]);
}
return map;
}
public static Map map(K[] keys, V[] values) {
Map map = new LinkedHashMap<>(keys.length);
int index = 0;
for (K key : keys) {
if (index < keys.length) {
V value = values[index];
map.put(key, value);
} else {
map.put(key, null);
}
index++;
}
return map;
}
@SafeVarargs
public static NavigableSet safeSortedSet(V... array) {
NavigableSet set = new ConcurrentSkipListSet();
for (int i = 0; i < array.length; i++) {
V v = array[i];
set.add(v);
}
return set;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy