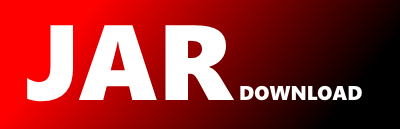
com.tdder.junit.jupiter.extension.TeardownExtension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of junit-teardown-extension Show documentation
Show all versions of junit-teardown-extension Show documentation
JUnit Jupiter extension. Provides Automated Teardown mechanism.
The newest version!
package com.tdder.junit.jupiter.extension;
import java.lang.reflect.Executable;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.List;
import java.util.function.Predicate;
import org.junit.jupiter.api.extension.AfterAllCallback;
import org.junit.jupiter.api.extension.AfterEachCallback;
import org.junit.jupiter.api.extension.BeforeAllCallback;
import org.junit.jupiter.api.extension.BeforeEachCallback;
import org.junit.jupiter.api.extension.ExtensionContext;
import org.junit.jupiter.api.extension.ParameterContext;
import org.junit.jupiter.api.extension.ParameterResolutionException;
import org.junit.jupiter.api.extension.ParameterResolver;
import org.junit.jupiter.api.extension.TestInstances;
import org.junit.platform.commons.support.HierarchyTraversalMode;
import org.junit.platform.commons.support.ModifierSupport;
import org.junit.platform.commons.support.ReflectionSupport;
/**
* JUnit Jupiter extension that tears down test fixtures.
*
* This extension is used with {@link TeardownRegistry}.
* When test adds teardown object to {@link TeardownRegistry}, added teardown object will be executed after test.
*
*
* Example:
*
*
* @ExtendWith(TeardownExtension.class)
* class MyTest {
*
* // This field is injected by TeardownExtension.
* private TeardownRegistry teardownRegistry;
*
* @Test
* void someTest() {
* // === Setup ===
*
* // Create test fixture
* final FooFixture fooFixture = createFooFixture(...);
*
* // Register tear down code block
* teardownRegistry.add(() -> fooFixture.clear());
*
* final BarFixture barFixture = createBarFixture(...);
* teardownRegistry.add(() -> barFixture.close());
*
* // ...
*
* // === Exercise ===
* // ...
*
* // === Verify ===
* // ...
*
* // After tests, either succeeded or failure, all added to TeardownRegistry code blocks are executed.
* // The execution order is in reverse order of addition to the TeardownRegistry.
* }
*
* }
*
*
* @see TeardownRegistry
* @author manhole
*/
public class TeardownExtension
implements ParameterResolver, BeforeAllCallback, AfterAllCallback, BeforeEachCallback, AfterEachCallback {
private static final ExtensionContext.Namespace NAMESPACE = ExtensionContext.Namespace.create(
TeardownExtension.class);
private final Object INSTANCE_STORE_KEY = TeardownExtension.class.getName() + "_INSTANCE";
private final Object STATIC_STORE_KEY = TeardownExtension.class.getName() + "_STATIC";
@Override
public boolean supportsParameter(final ParameterContext parameterContext, final ExtensionContext extensionContext)
throws ParameterResolutionException {
final boolean method = parameterContext.getDeclaringExecutable() instanceof Method;
return parameterContext.getParameter().getType() == TeardownRegistry.class && method;
}
@Override
public Object resolveParameter(final ParameterContext parameterContext, final ExtensionContext extensionContext)
throws ParameterResolutionException {
final Executable executable = parameterContext.getDeclaringExecutable();
if (ModifierSupport.isStatic(executable)) {
// @BeforeAll
return resolveParameter(extensionContext, STATIC_STORE_KEY);
} else {
// @Before, test method
return resolveParameter(extensionContext, INSTANCE_STORE_KEY);
}
}
public Object resolveParameter(final ExtensionContext extensionContext, final Object storeKey)
throws ParameterResolutionException {
final ExtensionContext.Store store = extensionContext.getStore(NAMESPACE);
return store.getOrComputeIfAbsent(storeKey, (v) -> new TeardownRegistryImpl(), TeardownRegistryImpl.class);
}
@Override
public void beforeAll(final ExtensionContext extensionContext) throws Exception {
injectStaticFields(extensionContext);
}
@Override
public void beforeEach(final ExtensionContext extensionContext) throws Exception {
final TestInstances requiredTestInstances = extensionContext.getRequiredTestInstances();
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy