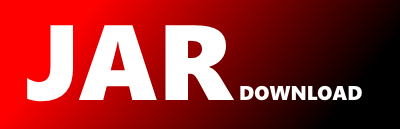
org.conqat.engine.index.shared.tests.TestTestExecutionWithPartitionBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons-test-fixtures Show documentation
Show all versions of teamscale-commons-test-fixtures Show documentation
Provides common DTOs for Teamscale
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.index.shared.tests;
import static com.teamscale.commons.commit.CommitDescriptorTestUtils.masterCommit;
import java.time.Duration;
import java.util.ArrayList;
import java.util.List;
import org.conqat.engine.index.shared.CommitDescriptor;
import org.conqat.engine.index.shared.ParentedCommitDescriptor;
/** Builder for creating {@link TestExecutionWithPartition}s for testing. */
public class TestTestExecutionWithPartitionBuilder {
private String uniformPath;
private long durationSeconds;
private ETestExecutionResult result;
private String message;
private CommitDescriptor commit;
private String partition;
private boolean isArtificialMergeTestExecution;
private List predecessorCommits = new ArrayList<>();
/**
* Creates a {@link TestTestExecutionWithPartitionBuilder} with some default
* values for the {@link TestExecutionWithPartition}.
*/
public static TestTestExecutionWithPartitionBuilder someTestExecution() {
return new TestTestExecutionWithPartitionBuilder()//
.withUniformPath("TestClass/testCase")//
.withDurationSeconds(1)//
.withResult(ETestExecutionResult.PASSED)//
.withMessage(null)//
.withCommit(masterCommit(1))//
.withPartition("Unit Tests")//
.withIsArtificialMergeTestExecution(false);
}
private TestTestExecutionWithPartitionBuilder() {
// Intentionally private. Clients must obtain Builder via someTestExecution().
}
/** Sets {@link #uniformPath}. */
public TestTestExecutionWithPartitionBuilder withUniformPath(String uniformPath) {
this.uniformPath = uniformPath;
return this;
}
/** Sets {@link #durationSeconds}. */
public TestTestExecutionWithPartitionBuilder withDurationSeconds(long durationSeconds) {
this.durationSeconds = durationSeconds;
return this;
}
/** Sets {@link #result}. */
public TestTestExecutionWithPartitionBuilder withResult(ETestExecutionResult result) {
this.result = result;
return this;
}
/** Sets {@link #message}. */
public TestTestExecutionWithPartitionBuilder withMessage(String message) {
this.message = message;
return this;
}
/**
* Sets {@link #commit} as well as the {@link #predecessorCommits} if the commit
* is a {@link ParentedCommitDescriptor}.
*/
public TestTestExecutionWithPartitionBuilder withCommit(CommitDescriptor commit) {
this.commit = commit;
if (commit instanceof ParentedCommitDescriptor) {
predecessorCommits.addAll(((ParentedCommitDescriptor) commit).getParentCommits());
}
return this;
}
/** Sets {@link #partition}. */
public TestTestExecutionWithPartitionBuilder withPartition(String partition) {
this.partition = partition;
return this;
}
/** Sets {@link #isArtificialMergeTestExecution}. */
public TestTestExecutionWithPartitionBuilder withIsArtificialMergeTestExecution(
boolean isArtificialMergeTestExecution) {
this.isArtificialMergeTestExecution = isArtificialMergeTestExecution;
return this;
}
/** Returns the built {@link TestExecutionWithPartition}. */
public TestExecutionWithPartition build() {
TestExecution testExecution = TestExecution.Builder//
.fromPath(uniformPath)//
.setResult(result)//
.setDuration(Duration.ofSeconds(durationSeconds))//
.setFailureMessage(message)//
.build();
return new TestExecutionWithPartition(testExecution, partition, commit, predecessorCommits,
isArtificialMergeTestExecution);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy