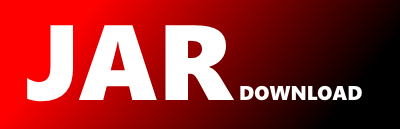
com.teamscale.commons.links.TeamscaleCommitLinkProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.teamscale.commons.links;
import static org.conqat.lib.commons.string.StringUtils.EMPTY_STRING;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.engine.index.shared.CommitDescriptor;
import org.conqat.engine.index.shared.PublicProjectId;
import org.conqat.lib.commons.net.UrlUtils;
import org.conqat.lib.commons.region.LineBasedRegion;
import org.conqat.lib.commons.region.OffsetBasedRegion;
import com.teamscale.commons.service.client.ServiceClientUris;
/**
* Helper class for obtaining links to global and project specific services of the current Teamscale
* instance. If a non-null {@link CommitDescriptor} is passed, all project services supporting the
* {@link ServiceClientUris#TIMESTAMP_PARAMETER_NAME} use this commit for queries.
*/
public class TeamscaleCommitLinkProvider extends TeamscaleProjectLinkProvider {
private final @Nullable CommitDescriptor commit;
/**
* Constructor.
*
* @param commit
* The commit that we should link to. Should be an exact commit, not a HEAD commit. The
* {@link TeamscaleCommitLinkProvider} will use a HEAD link where it makes sense
* automatically.
*/
public TeamscaleCommitLinkProvider(String baseUrl, PublicProjectId projectId, @Nullable CommitDescriptor commit) {
super(baseUrl, projectId);
this.commit = commit;
}
/**
* Returns a link to the findings detail view for the given finding, pointing to the finding at the
* HEAD of the branch.
*/
public String createFindingsDetailLink(String findingId) {
return baseUrl + "findings/details/" + UrlUtils.encodePathSegment(projectId.toString())
+ createFindingIdOptionString(findingId);
}
/** Returns a link to the activity details view for the current commit. */
public String createCommitDetailsLink() {
return baseUrl + "activity/details/" + UrlUtils.encodePathSegment(projectId.toString())
+ createCommitOptionString();
}
/** Returns a link to the activity details view for the current commit. */
public String createCommitAlertDetailsLink(CommitDescriptor commit) {
return baseUrl + "activity/details/" + UrlUtils.encodePathSegment(projectId.toString())
+ createCommitOptionString(commit);
}
/** Returns a link to the file code view. */
public String createTestGapDetailsLink(String uniformPath, int methodStartOffset, int methodEndOffset) {
return baseUrl + "metrics/code/" + UrlUtils.encodePathSegment(projectId.toString()) + "/"
+ UrlUtils.encodePathSegment(uniformPath)
+ createCodeViewOptionString(methodStartOffset, methodEndOffset);
}
private String createCodeViewOptionString(int methodStartOffset, int methodEndOffset) {
return ServiceClientUris.createOptionString(ServiceClientUris.TIMESTAMP_PARAMETER_NAME,
CommitDescriptor.latestOnBranch(commit.getBranchName()).toServiceCallFormat(), "selection",
"char-" + methodStartOffset + "-" + methodEndOffset);
}
/** Returns a link to the code view for the given file and region. */
public String createLinkToFile(String uniformPath, OffsetBasedRegion region) {
return createLinkToFile(uniformPath, "char-" + region.getStart() + "-" + region.getEnd());
}
/** Returns a link to the code view for the given file and region. */
public String createLinkToFile(String uniformPath, LineBasedRegion lineBasedRegion) {
return createLinkToFile(uniformPath, lineBasedRegion.getStart() + "-" + lineBasedRegion.getEnd());
}
private String createLinkToFile(String uniformPath, String selection) {
String optionString;
if (commit == null) {
optionString = ServiceClientUris.createOptionString("selection", selection);
} else {
optionString = ServiceClientUris.createOptionString(ServiceClientUris.TIMESTAMP_PARAMETER_NAME,
commit.toServiceCallFormat(), "selection", selection);
}
return baseUrl + "metrics/code/" + UrlUtils.encodePathSegment(projectId.toString()) + "/"
+ UrlUtils.encodePathSegment(uniformPath) + optionString;
}
private String createCommitOptionString() {
return createCommitOptionString(commit);
}
private static String createCommitOptionString(CommitDescriptor commit) {
if (commit == null) {
return EMPTY_STRING;
}
return ServiceClientUris.createOptionString(ServiceClientUris.TIMESTAMP_PARAMETER_NAME,
commit.toServiceCallFormat());
}
private String createFindingIdOptionString(String findingId) {
if (commit == null) {
return ServiceClientUris.createOptionString("id", findingId);
}
return ServiceClientUris.createOptionString("id", findingId, ServiceClientUris.TIMESTAMP_PARAMETER_NAME,
CommitDescriptor.latestOnBranch(commit.getBranchName()).toServiceCallFormat());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy