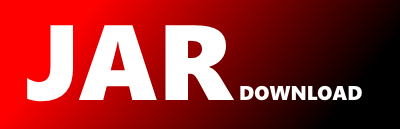
com.teamscale.commons.links.TeamscaleLinkProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.teamscale.commons.links;
import static org.conqat.lib.commons.net.UrlUtils.encodeQueryParameter;
import static org.conqat.lib.commons.string.StringUtils.EMPTY_STRING;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.lib.commons.assessment.ETrafficLightColor;
import org.conqat.lib.commons.string.StringUtils;
/**
* Helper class for obtaining links to global services of the current Teamscale instance.
*/
public class TeamscaleLinkProvider {
/** The base URL of the Teamscale instance. */
protected final String baseUrl;
public TeamscaleLinkProvider(String baseUrl) {
if (StringUtils.isEmpty(baseUrl)) {
baseUrl = "https://no-teamscale-base-url-configured-in-teamscale-server-options.com";
}
this.baseUrl = StringUtils.ensureEndsWith(baseUrl, "/");
}
/** Returns the URL for the static findings badge. */
public String createStaticFindingsBadgeUrl(int addedFindings, int inChangedCodeFindings, int removedFindings) {
String badgeServiceUrl = "api/badges/findings/static";
return baseUrl + badgeServiceUrl + "?added=" + addedFindings + "&changed=" + inChangedCodeFindings + "&removed="
+ removedFindings;
}
/** Returns the URL for the static spec items badge. */
public String createStaticSpecItemsBadgeUrl(int affectedSpecItems) {
String badgeServiceUrl = "api/badges/spec-items/static";
return baseUrl + badgeServiceUrl + "?affectedItems=" + affectedSpecItems;
}
/** Returns the URL for the static test gap badge. */
public String createStaticTgaBadgeUrl(double ratio, int testedChurn, int untestedChange, int untestedAddition,
@Nullable String incompleteBuildWarningText) {
String badgeServiceUrl = "api/badges/test-gap/static";
String staticTgaBadgeUrl = baseUrl + badgeServiceUrl + "?ratio=" + ratio + "&testedChurn=" + testedChurn
+ "&untestedChange=" + untestedChange + "&untestedAddition=" + untestedAddition + "&format=assessment";
if (!StringUtils.isEmpty(incompleteBuildWarningText)) {
staticTgaBadgeUrl += "&incompleteBuildText=" + incompleteBuildWarningText;
}
return staticTgaBadgeUrl;
}
/** @return the URL for the static metric badge. */
public String createStaticMetricBadgeUrl(String metricName, @Nullable ETrafficLightColor thresholdAssessment,
String metricValue, @Nullable String incompleteBuildWarningText) {
String badgeServiceUrl = "api/badges/metric/static";
String colorParameter = EMPTY_STRING;
if (thresholdAssessment != null) {
colorParameter = "&color=" + encodeQueryParameter(thresholdAssessment.name());
}
String staticMetricThresholdBadgeUrl = baseUrl + badgeServiceUrl + "?metric=" + encodeQueryParameter(metricName)
+ colorParameter + "&value=" + encodeQueryParameter(metricValue);
if (!StringUtils.isEmpty(incompleteBuildWarningText)) {
staticMetricThresholdBadgeUrl += "&incompleteBuildText=" + encodeQueryParameter(incompleteBuildWarningText);
}
return staticMetricThresholdBadgeUrl;
}
/** Returns the URL for the static commit alerts icon badge. */
public String createStaticCommitAlertsBadgeUrl(int commitAlerts) {
String badgeServiceUrl = "api/badges/commit-alerts/static";
return baseUrl + badgeServiceUrl + "?commitAlerts=" + commitAlerts;
}
/**
* Returns a link to the Teamscale logo (with gray 'Teamscale' text).
*/
public String createTeamscaleLogoLink() {
return baseUrl + "images/teamscale-logo-gray-text-with-margin.svg";
}
/**
* Returns a link to the Teamscale logo (with gray 'Teamscale' text) to be specifically used for
* pull requests in Bitbucket Server. This is used to ensure that the Teamscale logo is correctly
* aligned with the finding badge in that specific case.
*/
public String createTeamscaleLogoLinkForBitbucketServer() {
return baseUrl + "images/teamscale-logo-gray-text-bitbucket.png";
}
/**
* Returns a link to the Teamscale logo without text. We use a PNG here (no SVG) as this is
* guaranteed to be rendered in the correct size.
*/
public String createTeamscaleNotificationLogoLink() {
return baseUrl + "images/notification-logo.png";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy