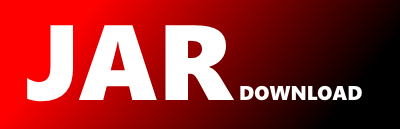
com.teamscale.commons.service.ServiceUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.teamscale.commons.service;
import java.io.File;
import java.net.URISyntaxException;
import java.util.Optional;
import java.util.function.Function;
import org.conqat.lib.commons.net.UrlUtils;
import org.conqat.lib.commons.string.StringUtils;
/**
* Utility methods for services.
*/
public class ServiceUtils {
/** Uses Java API to check if the server address is valid */
public static boolean isValidServerAddress(String address) {
// Skip further checks if the path refers to an existing directory
if (new File(address).exists()) {
return true;
}
// Ensure that some kind of protocol is used
if (!address.contains("://")) {
return false;
}
try {
// Check whether the given address is a valid URI.
UrlUtils.parseUri(address);
} catch (URISyntaxException e) {
return false;
}
return true;
}
/**
* Produces a specific error message if the identifier is invalid (e.g., contains linebreaks,
* invisible unicode chars, or is empty). The error message contains the (truncated) text if it is
* not empty.
*
* @param identifierText
* the text of the identifier (e.g., "x" or "foo")
* @param identifierDescription
* a description of what this identifier should be (e.g., "Group name" or "Id"). This is
* used at the beginning of the error message and therefore should start with an
* uppercase letter.
*/
public static Optional getErrorMessageForInvalidIdentifiers(String identifierText,
String identifierDescription) {
Function truncate = identifier -> "\""
+ StringUtils.removeAll(StringUtils.truncateWithThreeDots(identifier, 9), "\n") + "\"";
if (identifierText.contains("\n")) {
return Optional.of(identifierDescription + " " + truncate.apply(identifierText) + " contains linebreaks");
}
if (identifierText.matches(".*\\p{C}.*")) {
return Optional.of(identifierDescription + " " + truncate.apply(identifierText)
+ " contains invisible Unicode control characters");
}
if (identifierText.equals(StringUtils.EMPTY_STRING)) {
return Optional.of(identifierDescription + " is empty");
}
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy