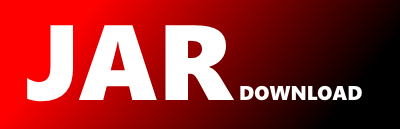
com.teamscale.commons.service.client.IDeserializationFormat Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.teamscale.commons.service.client;
import java.io.IOException;
import java.io.Reader;
import java.util.List;
import java.util.Optional;
import org.conqat.engine.commons.util.JsonSerializationException;
import org.conqat.engine.commons.util.JsonUtils;
import org.conqat.lib.commons.function.FunctionWithException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.JavaType;
import com.google.common.io.CharStreams;
import com.teamscale.commons.service.EMimeType;
/**
* Provides a deserialization strategy for service calls.
*/
public interface IDeserializationFormat {
/**
* Returns the MIME type that should be sent to the server as "Accept" header. May return empty to
* indicate that the Accept header should not be set.
*/
Optional getMimeType();
/** Deserializes the given data to an object. */
T deserialize(Reader serializedData) throws IOException;
/** Constructs a deserializer for the given data type from JSON. */
static IDeserializationFormat fromJson(Class elementType) {
return fromJson(serializedData -> JsonUtils.deserializeFromJson(serializedData, elementType));
}
/** Constructs a deserializer for the given (complex) data type from JSON. */
static IDeserializationFormat fromJson(TypeReference elementType) {
return fromJson(serializedData -> JsonUtils.deserializeFromJson(serializedData, elementType));
}
/** Constructs a deserializer for a list of the given data type from JSON. */
static IDeserializationFormat> fromJsonList(Class elementType) {
JavaType listType = JsonUtils.getJavaListType(elementType);
return fromJson(serializedData -> JsonUtils.deserializeFromJson(serializedData, listType));
}
/**
* Constructs a JSON {@link IDeserializationFormat} using the provided {@code deserializer} for
* deserializing the JSON data.
*/
static IDeserializationFormat fromJson(
FunctionWithException deserializer) {
return new IDeserializationFormat<>() {
@Override
public Optional getMimeType() {
return Optional.of(EMimeType.JSON);
}
@Override
public T deserialize(Reader serializedData) throws IOException {
try {
return deserializer.apply(serializedData);
} catch (JsonSerializationException e) {
throw new IOException(e.getMessage(), e);
}
}
};
}
/** Constructs a deserializer for plain text responses. */
static IDeserializationFormat asPlainText() {
return new IDeserializationFormat<>() {
@Override
public Optional getMimeType() {
return Optional.of(EMimeType.PLAIN);
}
@Override
public String deserialize(Reader serializedData) throws IOException {
return CharStreams.toString(serializedData);
}
};
}
/**
* Constructs a deserializer for a request without setting the Accept header. The response is
* returned as a plain string.
*/
static IDeserializationFormat asUnspecifiedPlainText() {
return new IDeserializationFormat<>() {
@Override
public Optional getMimeType() {
return Optional.empty();
}
@Override
public String deserialize(Reader serializedData) throws IOException {
return CharStreams.toString(serializedData);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy