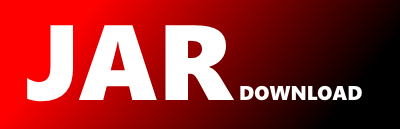
com.teamscale.commons.service.client.ServiceClientUris Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.teamscale.commons.service.client;
import static org.conqat.lib.commons.collections.ListMap.fromNonEmptyPairs;
import java.util.List;
import org.conqat.engine.index.shared.CommitDescriptor;
import org.conqat.engine.index.shared.PublicProjectId;
import org.conqat.lib.commons.collections.ListMap;
import org.conqat.lib.commons.collections.Pair;
import org.conqat.lib.commons.net.UrlUtils;
import org.conqat.lib.commons.string.StringUtils;
import org.conqat.lib.commons.uniformpath.UniformPath;
import org.conqat.lib.commons.version.Version;
/** Methods to build Teamscale service URIs. */
public class ServiceClientUris {
/** Name of the boolean query parameter used to indicate recursive queries. */
public static final String RECURSIVE_PARAMETER = "recursive";
/** Baselines parameter for findings service. */
public static final String BASELINE_PARAMETER = "baseline";
/** Include changed code findings parameter. */
public static final String INCLUDE_CHANGED_CODE_FINDINGS_PARAMETER = "include-changed-code-findings";
/** View only changed code findings parameter. */
public static final String ONLY_CHANGED_CODE_FINDINGS_PARAMETER = "only-changed-code-findings";
/** Principal metric index parameter for metric distribution service */
public static final String PRINCIPAL_METRIC_INDEX_PARAMETER = "principal-metric-index";
/** Metric index parameter for metric distribution service */
public static final String METRIC_INDEXES_PARAMETER = "metric-indexes";
/** Boundaries parameter for metric distribution service */
public static final String BOUNDARY_PARAMETER = "boundaries";
/** Name of the timestamp parameter. */
public static final String TIMESTAMP_PARAMETER_NAME = "t";
private ServiceClientUris() {
// Utils class should not be instantiated
}
/** Constructs a global service uri for services with api prefix. */
public static String getGlobal(String serviceName, ServerDetails serverDetails, Pair, ?>... options) {
return getGlobal(serviceName, serverDetails, fromNonEmptyPairs(options));
}
/** Constructs a global service uri for services with api prefix and version. */
public static String getGlobal(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
Pair, ?>... options) {
return getGlobal(serviceName, serverDetails, teamscaleVersion, fromNonEmptyPairs(options));
}
/** Constructs a global service uri for services with api prefix and version. */
public static String getGlobal(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
ListMap options) {
return StringUtils.ensureEndsWith(serverDetails.getUrl(), "/") + "api/v" + teamscaleVersion + "/" + serviceName
+ createOptionString(options);
}
/** Constructs a global service uri for the given resource. */
public static String getGlobalResource(String resourcePath, ServerDetails serverDetails) {
return StringUtils.ensureEndsWith(serverDetails.getUrl(), "/") + resourcePath;
}
/** Constructs a global service uri for services with api prefix. */
public static String getGlobal(String serviceName, ServerDetails serverDetails, ListMap options) {
return StringUtils.ensureEndsWith(serverDetails.getUrl(), "/") + "api/" + serviceName
+ createOptionString(options);
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, PublicProjectId projectId,
Pair, ?>... options) {
return getProject(serviceName, serverDetails, projectId, fromNonEmptyPairs(options));
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
PublicProjectId projectId, Pair, ?>... options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, fromNonEmptyPairs(options));
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, PublicProjectId projectId,
ListMap options) {
return getProject(serviceName, serverDetails, projectId, StringUtils.EMPTY_STRING, options);
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
PublicProjectId projectId, ListMap options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, StringUtils.EMPTY_STRING, options);
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, PublicProjectId projectId,
UniformPath uniformPath, Pair, ?>... options) {
return getProject(serviceName, serverDetails, projectId, uniformPath.toString(), options);
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
PublicProjectId projectId, UniformPath uniformPath, Pair, ?>... options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, uniformPath.toString(), options);
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, PublicProjectId projectId,
String uniformPath, Pair, ?>... options) {
return getProject(serviceName, serverDetails, projectId, uniformPath, fromNonEmptyPairs(options));
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
PublicProjectId projectId, String uniformPath, Pair, ?>... options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, uniformPath,
fromNonEmptyPairs(options));
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, PublicProjectId projectId,
UniformPath uniformPath, ListMap options) {
return getProject(serviceName, serverDetails, projectId, uniformPath.toString(), options);
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
PublicProjectId projectId, UniformPath uniformPath, ListMap options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, uniformPath.toString(), options);
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, PublicProjectId projectId,
String uniformPath, ListMap options) {
return StringUtils.ensureEndsWith(serverDetails.getUrl(), "/") + "api/projects/" + projectId + "/" + serviceName
+ "/" + UrlUtils.encodePathSegment(uniformPath) + createOptionString(options);
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
PublicProjectId projectId, String uniformPath, ListMap options) {
return StringUtils.ensureEndsWith(serverDetails.getUrl(), "/") + "api/v" + teamscaleVersion + "/projects/"
+ projectId + "/" + serviceName + "/" + UrlUtils.encodePathSegment(uniformPath)
+ createOptionString(options);
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, String projectId,
Pair, ?>... options) {
return getProject(serviceName, serverDetails, projectId, fromNonEmptyPairs(options));
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
String projectId, Pair, ?>... options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, fromNonEmptyPairs(options));
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, String projectId,
ListMap options) {
return getProject(serviceName, serverDetails, projectId, StringUtils.EMPTY_STRING, options);
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
String projectId, ListMap options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, StringUtils.EMPTY_STRING, options);
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, String projectId,
UniformPath uniformPath, Pair, ?>... options) {
return getProject(serviceName, serverDetails, projectId, uniformPath.toString(), options);
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
String projectId, UniformPath uniformPath, Pair, ?>... options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, uniformPath.toString(), options);
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, String projectId,
String uniformPath, Pair, ?>... options) {
return getProject(serviceName, serverDetails, projectId, uniformPath, fromNonEmptyPairs(options));
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
String projectId, String uniformPath, Pair, ?>... options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, uniformPath,
fromNonEmptyPairs(options));
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, String projectId,
UniformPath uniformPath, ListMap options) {
return getProject(serviceName, serverDetails, projectId, uniformPath.toString(), options);
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
String projectId, UniformPath uniformPath, ListMap options) {
return getProject(serviceName, serverDetails, teamscaleVersion, projectId, uniformPath.toString(), options);
}
/** Constructs a project service uri for services with api prefix. */
public static String getProject(String serviceName, ServerDetails serverDetails, String projectId,
String uniformPath, ListMap options) {
return StringUtils.ensureEndsWith(serverDetails.getUrl(), "/") + "api/projects/"
+ UrlUtils.encodePathSegment(projectId) + "/" + serviceName + "/"
+ UrlUtils.encodePathSegment(uniformPath) + createOptionString(options);
}
/**
* Constructs a project service uri for services with api prefix and version.
*/
public static String getProject(String serviceName, ServerDetails serverDetails, Version teamscaleVersion,
String projectId, String uniformPath, ListMap options) {
return StringUtils.ensureEndsWith(serverDetails.getUrl(), "/") + "api/v" + teamscaleVersion + "/projects/"
+ UrlUtils.encodePathSegment(projectId) + "/" + serviceName + "/"
+ UrlUtils.encodePathSegment(uniformPath) + createOptionString(options);
}
/**
* Creates an encoded URL options string starting with ?
from the given options. The
* given parameters are interpreted as parameter names and values, where each odd numbered parameter
* is a parameter name and each even one is the corresponding value.
*/
public static String createOptionString(String... parametersAndValues) {
return createOptionString(ListMap.of(parametersAndValues));
}
/**
* Creates an encoded URL options string starting with the character ? from the given options.
*/
private static String createOptionString(ListMap options) {
StringBuilder sb = new StringBuilder();
if (options != null) {
String separator = "?";
for (String option : options.getKeys()) {
List values = options.getCollection(option);
for (String value : values) {
value = UrlUtils.encodeQueryParameter(value);
sb.append(separator).append(option).append('=').append(value);
separator = "&";
}
}
}
return sb.toString();
}
/**
* Creates a timestamp parameter pair. When the timestamp is null the method returns {@code null}.
*/
public static Pair createCommitDescriptorOption(CommitDescriptor commitDescriptor) {
if (commitDescriptor == null) {
return null;
}
return Pair.createPair(TIMESTAMP_PARAMETER_NAME, commitDescriptor.toServiceCallFormat());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy