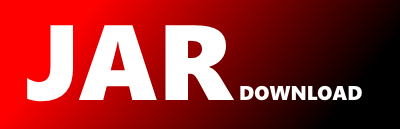
com.teamscale.commons.utils.ParameterFileUtils Maven / Gradle / Ivy
Show all versions of teamscale-commons Show documentation
package com.teamscale.commons.utils;
import java.util.Set;
import org.conqat.lib.commons.collections.Pair;
/**
* Utility methods concerning parameter files used for checks and metadata files for various
* languages. A parameter file defines on each line a parameter and its value, both separated by a
* colon.
*/
public class ParameterFileUtils {
private static final char COLON = ':';
/**
* Parses the given line from a parameters file and returns the parameter name and value. Throws an
* AssertionError if the parameter name does not match any name in the given optionNames set.
*
* This method works around the problem that colon (:) is the separator between option name and
* value, but colon is also allowed as part of the option names and as part of the option values.
*
* This method scans the colon signs from left to right and returns the first possible option name
* that occurs in the given set.
*
* For example, given the line "Empty blocks: allow empty constructors:true", this method returns
* new Pair("Empty blocks: allow empty constructors", "true")
.
*
* @param line
* the full line from a parameters file
* @param optionNames
* all potential option names
* @return parameter name and value
*/
public static Pair findParameterNameAndValue(String line, Set optionNames) {
int colonPosition = line.indexOf(COLON);
while (colonPosition != -1) {
String parameterName = line.substring(0, colonPosition).trim();
if (optionNames.contains(parameterName)) {
String parameterValue = line.substring(colonPosition + 1).trim().replace("\\n", "\n");
return new Pair<>(parameterName, parameterValue);
}
colonPosition = line.indexOf(COLON, colonPosition + 1);
}
return null;
}
}