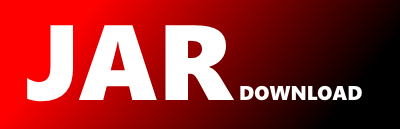
com.teamscale.index.findings.calculation.FindingsWithCount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.teamscale.index.findings.calculation;
import static java.util.Objects.requireNonNull;
import java.util.List;
import org.conqat.engine.index.shared.TrackedFinding;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.Preconditions;
/**
* Helper object for adding count information to the actual list of findings.
*
* This class is used as DTO during communication with IDE clients via
* {@link com.teamscale.ide.commons.client.IIdeServiceClient}, special care has to be taken when
* changing its signature!
*/
public class FindingsWithCount {
/** The name of the JSON property name for {@link #findings}. */
public static final String FINDINGS_PROPERTY = "findings";
/**
* The name of the JSON property name for {@link #nonBlacklistedFindingsCount}.
*/
public static final String NON_BLACKLISTED_FINDINGS_COUNT_PROPERTY = "nonBlacklistedFindingsCount";
/** The name of the JSON property name for {@link #toleratedFindingsCount}. */
public static final String TOLERATED_FINDINGS_COUNT_PROPERTY = "toleratedFindingsCount";
/** The name of the JSON property name for {@link #falsePositivesCount}. */
public static final String FALSE_POSITIVES_COUNT_PROPERTY = "falsePositivesCount";
/** The findings. */
@JsonProperty(FINDINGS_PROPERTY)
private List findings;
/** The number of non-blacklisted findings. */
@JsonProperty(NON_BLACKLISTED_FINDINGS_COUNT_PROPERTY)
private final int nonBlacklistedFindingsCount;
/** The number of ignored findings. */
@JsonProperty(TOLERATED_FINDINGS_COUNT_PROPERTY)
private final int toleratedFindingsCount;
/** The number of false-positives. */
@JsonProperty(FALSE_POSITIVES_COUNT_PROPERTY)
private final int falsePositivesCount;
@JsonCreator
public FindingsWithCount(@JsonProperty(FINDINGS_PROPERTY) List findings,
@JsonProperty(NON_BLACKLISTED_FINDINGS_COUNT_PROPERTY) int nonBlacklistedFindingsCount,
@JsonProperty(TOLERATED_FINDINGS_COUNT_PROPERTY) int toleratedFindingsCount,
@JsonProperty(FALSE_POSITIVES_COUNT_PROPERTY) int falsePositivesCount) {
Preconditions.checkArgument(nonBlacklistedFindingsCount >= 0);
Preconditions.checkArgument(toleratedFindingsCount >= 0);
Preconditions.checkArgument(falsePositivesCount >= 0);
this.findings = requireNonNull(findings);
this.nonBlacklistedFindingsCount = nonBlacklistedFindingsCount;
this.toleratedFindingsCount = toleratedFindingsCount;
this.falsePositivesCount = falsePositivesCount;
}
/** @see #findings */
public List getFindings() {
return findings;
}
/**
* Returns the number of non-blacklisted findings. As opposed to {@link #getFindings()} this is
* before filtering/truncation.
*/
public int getNonBlacklistedFindingsCount() {
return nonBlacklistedFindingsCount;
}
/**
* Returns the number of tolerated findings. As opposed to {@link #getFindings()} this is before
* filtering/truncation.
*/
public int getToleratedFindingsCount() {
return toleratedFindingsCount;
}
/**
* Returns the number of false positive findings. As opposed to {@link #getFindings()} this is
* before filtering/truncation.
*/
public int getFalsePositivesCount() {
return falsePositivesCount;
}
public void setFindings(List adjustedFindings) {
findings = adjustedFindings;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy