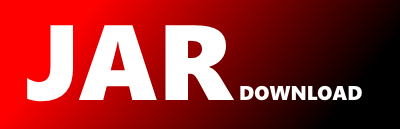
com.teamscale.index.metadata.abap.AbapFileMetadata Maven / Gradle / Ivy
Show all versions of teamscale-commons Show documentation
package com.teamscale.index.metadata.abap;
import java.io.Serializable;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.lib.commons.collections.CounterSet;
import org.conqat.lib.commons.collections.PairList;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.teamscale.index.metadata.IFileMetadata;
/**
* Collects various information about an ABAP object, from an ABAP full or incremental export zip
* file, that will be persisted and used for analysis in Teamscale.
*/
@IndexValueClass
public class AbapFileMetadata implements IFileMetadata {
private static final long serialVersionUID = 1L;
private static final String FILE_PATH_PROPERTY = "filePath";
/**
* Originating system property. This id must not be changed, it is used in the .metadata files
* stored in the Abap git.
*/
public static final String ORIGINATING_SYSTEM_PROPERTY = "originatingSystem";
/**
* Creation date property. This id must not be changed, it is used in the .metadata files stored in
* the Abap git.
*/
public static final String CREATION_DATE_PROPERTY = "creationDate";
/**
* Last update date/time property. This id must not be changed, it is used in the .metadata files
* stored in the Abap git.
*/
public static final String LAST_UPDATE_DATE_TIME_PROPERTY = "lastUpdateDateTime";
/**
* Program type property. This id must not be changed, it is used in the .metadata files stored in
* the Abap git.
*/
public static final String PROGRAM_TYPE_PROPERTY = "programType";
/**
* Authorization group property. This id must not be changed, it is used in the .metadata files
* stored in the Abap git.
*/
public static final String AUTHORIZATION_GROUP_PROPERTY = "authorizationGroup";
/**
* RFC Enablement property. This id must not be changed, it is used in the .metadata files stored in
* the Abap git.
*/
public static final String IS_RFC_ENABLED_PROPERTY = "isRfcEnabled";
/**
* Code generation property. This id must not be changed, it is used in the .metadata files stored
* in the Abap git.
*/
public static final String IS_GENERATED_CODE_PROPERTY = "isGeneratedCode";
/**
* ABAP language version property. This id must not be changed, it is used in the .metadata files
* stored in the Abap git.
*/
public static final String LANGUAGE_VERSION_PROPERTY = "languageVersion";
/**
* Release contract property. This id must not be changed, it is used in the .metadata files stored
* in the Abap git.
*/
public static final String RELEASE_CONTRACT_PROPERTY = "releaseContract";
/**
* Path to the file.
*/
@JsonProperty(FILE_PATH_PROPERTY)
private final String filePath;
/**
* The SAP system in which this ABAP object was created.
*/
@JsonProperty(ORIGINATING_SYSTEM_PROPERTY)
private @Nullable String originatingSystem;
/**
* The date at which this ABAP object was created.
*/
@JsonProperty(CREATION_DATE_PROPERTY)
private long creationDate;
/**
* The date and time at which an ABAP object was last modified.
*/
@JsonProperty(LAST_UPDATE_DATE_TIME_PROPERTY)
private long lastUpdateDateTime;
/**
* The type of program that must be defined at its creation e.g. Executable program, include
* program, e.t.c. Read docs
* for more information.
*/
@JsonProperty(PROGRAM_TYPE_PROPERTY)
private @Nullable EAbapProgramType programType;
/**
* Authorization group are objects that secure ABAP programs against unauthorized access e.g.
* S_PROGRAM. Read the following docs for more information.
*
*/
@JsonProperty(AUTHORIZATION_GROUP_PROPERTY)
private @Nullable String authorizationGroup;
/**
* Stores whether an ABAP object is enabled for RFC communication.
*/
@JsonProperty(IS_RFC_ENABLED_PROPERTY)
private boolean rfcIsEnabled;
/**
* Stores whether an ABAP object is generated. Read
* docs for
* more information.
*/
@JsonProperty(IS_GENERATED_CODE_PROPERTY)
private boolean codeIsGenerated;
/**
* Stores the ABAP language version of some ABAP object. See language
* version for SAP NetWeaver AS ABAP Release 752 and language
* version for newer SAP systems.
*/
@JsonProperty(LANGUAGE_VERSION_PROPERTY)
private @Nullable EAbapLanguageVersion languageVersion;
/**
* Stores release contract infos for some ABAP object.
*/
@JsonProperty(RELEASE_CONTRACT_PROPERTY)
private @Nullable ReleaseContract releaseContract;
/**
* Constructor to help create JSON objects.
*/
@JsonCreator
public AbapFileMetadata(@JsonProperty(FILE_PATH_PROPERTY) String filePath,
@JsonProperty(CREATION_DATE_PROPERTY) long creationDate,
@JsonProperty(LAST_UPDATE_DATE_TIME_PROPERTY) long lastUpdateDateTime,
@JsonProperty(ORIGINATING_SYSTEM_PROPERTY) @Nullable String originatingSystem,
@JsonProperty(PROGRAM_TYPE_PROPERTY) @Nullable EAbapProgramType programType,
@JsonProperty(AUTHORIZATION_GROUP_PROPERTY) @Nullable String authorizationGroup,
@JsonProperty(LANGUAGE_VERSION_PROPERTY) @Nullable EAbapLanguageVersion languageVersion,
@JsonProperty(RELEASE_CONTRACT_PROPERTY) @Nullable ReleaseContract releaseContract,
@JsonProperty(IS_RFC_ENABLED_PROPERTY) boolean rfcIsEnabled,
@JsonProperty(IS_GENERATED_CODE_PROPERTY) boolean codeIsGenerated) {
this.filePath = filePath;
this.creationDate = creationDate;
this.lastUpdateDateTime = lastUpdateDateTime;
this.originatingSystem = originatingSystem;
this.programType = programType;
this.authorizationGroup = authorizationGroup;
this.languageVersion = languageVersion;
this.releaseContract = releaseContract;
this.rfcIsEnabled = rfcIsEnabled;
this.codeIsGenerated = codeIsGenerated;
}
@Override
public PairList getMetrics() {
PairList metrics = new PairList<>();
metrics.add(CREATION_DATE_PROPERTY, creationDate);
metrics.add(LAST_UPDATE_DATE_TIME_PROPERTY, lastUpdateDateTime);
metrics.add(ORIGINATING_SYSTEM_PROPERTY, toMetric(originatingSystem));
metrics.add(PROGRAM_TYPE_PROPERTY, EAbapProgramType.toMetric(programType));
metrics.add(LANGUAGE_VERSION_PROPERTY, EAbapLanguageVersion.toMetric(languageVersion));
metrics.add(AUTHORIZATION_GROUP_PROPERTY, toMetric(authorizationGroup));
return metrics;
}
/**
* Transforms the string value into a matching {@link CounterSet}.
*
* We are using {@link CounterSet counter sets} because we don't lose the amount of files with the
* specific string on aggregation in a folder.
*/
private CounterSet toMetric(@Nullable String value) {
if (value == null) {
// CounterSets are not allowed to have null as key value
return CounterSet.empty();
} else {
return new CounterSet<>(value, 1);
}
}
/** Returns the {@link #filePath}. */
public String getFilePath() {
return filePath;
}
/** Returns the {@link #originatingSystem}. */
public String getOriginatingSystem() {
return originatingSystem;
}
/** Returns the creation date. */
public long getCreationDate() {
return creationDate;
}
/** Returns the last update date and time. */
public long getLastUpdateDateTime() {
return lastUpdateDateTime;
}
/** @see #originatingSystem */
public void setOriginatingSystem(String originatingSystem) {
this.originatingSystem = originatingSystem;
}
/** @see #lastUpdateDateTime */
public void setLastUpdateDateTime(long lastUpdateDateTime) {
this.lastUpdateDateTime = lastUpdateDateTime;
}
/** @see #creationDate */
public void setCreationDate(long creationDate) {
this.creationDate = creationDate;
}
/** @see #rfcIsEnabled */
public boolean isRfcEnabled() {
return rfcIsEnabled;
}
/** @see #rfcIsEnabled */
public void setRfcEnabled(boolean rfcIsEnabled) {
this.rfcIsEnabled = rfcIsEnabled;
}
/** @see #programType */
public void setProgramType(EAbapProgramType programType) {
this.programType = programType;
}
/** @see #languageVersion */
public void setLanguageVersion(EAbapLanguageVersion languageVersion) {
this.languageVersion = languageVersion;
}
/** @see #authorizationGroup */
public void setAuthorizationGroup(String authorizationGroup) {
this.authorizationGroup = authorizationGroup;
}
/** @see #releaseContract */
public void setReleaseContract(ReleaseContract releaseContract) {
this.releaseContract = releaseContract;
}
/** @see #codeIsGenerated */
public void setCodeIsGenerated(boolean codeIsGenerated) {
this.codeIsGenerated = codeIsGenerated;
}
/** @see #programType */
public EAbapProgramType getProgramType() {
return programType;
}
/** @see #languageVersion */
public EAbapLanguageVersion getLanguageVersion() {
return languageVersion;
}
/** @see #authorizationGroup */
public String getAuthorizationGroup() {
return authorizationGroup;
}
/** @see #releaseContract */
public ReleaseContract getReleaseContract() {
return releaseContract;
}
/** @see #codeIsGenerated */
public boolean isCodeGenerated() {
return codeIsGenerated;
}
}